如何在现有的深度学习模型CNN上增加自注意力机制模块,用python展示
时间: 2023-06-17 07:06:57 浏览: 51
在现有的CNN模型中增加自注意力机制模块,可以使用自注意力机制模块来增强模型对输入数据的关注力,从而提高模型性能。具体步骤如下:
1.导入需要的库
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
```
2.定义自注意力机制模块
```python
class SelfAttention(layers.Layer):
def __init__(self, embed_dim):
super(SelfAttention, self).__init__()
self.embed_dim = embed_dim
self.query_dense = layers.Dense(embed_dim)
self.key_dense = layers.Dense(embed_dim)
self.value_dense = layers.Dense(embed_dim)
self.combine_heads = layers.Dense(embed_dim)
def attention(self, query, key, value):
score = tf.matmul(query, key, transpose_b=True)
dim_key = tf.cast(tf.shape(key)[-1], tf.float32)
scaled_score = score / tf.math.sqrt(dim_key)
weights = tf.nn.softmax(scaled_score, axis=-1)
output = tf.matmul(weights, value)
return output, weights
def separate_heads(self, x, batch_size):
x = tf.reshape(x, (batch_size, -1, self.num_heads, self.head_dim))
return tf.transpose(x, perm=[0, 2, 1, 3])
def call(self, inputs):
batch_size = tf.shape(inputs)[0]
query = self.query_dense(inputs)
key = self.key_dense(inputs)
value = self.value_dense(inputs)
query = self.separate_heads(query, batch_size)
key = self.separate_heads(key, batch_size)
value = self.separate_heads(value, batch_size)
scaled_attention, weights = self.attention(query, key, value)
scaled_attention = tf.transpose(scaled_attention, perm=[0, 2, 1, 3])
concat_attention = tf.reshape(scaled_attention, (batch_size, -1, self.embed_dim))
output = self.combine_heads(concat_attention)
return output
```
3.定义CNN模型并增加自注意力机制模块
```python
inputs = keras.Input(shape=(28, 28, 1))
x = layers.Conv2D(64, 3, activation="relu")(inputs)
x = SelfAttention(64)(x) # 增加自注意力机制模块
x = layers.MaxPooling2D(2)(x)
x = layers.Conv2D(128, 3, activation="relu")(x)
x = SelfAttention(128)(x) # 增加自注意力机制模块
x = layers.MaxPooling2D(2)(x)
x = layers.Conv2D(256, 3, activation="relu")(x)
x = layers.Flatten()(x)
x = layers.Dense(256, activation="relu")(x)
outputs = layers.Dense(10)(x)
model = keras.Model(inputs=inputs, outputs=outputs)
```
4.训练模型
```python
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
```
通过增加自注意力机制模块,可以提高模型的性能,从而更好地适应数据集。
相关推荐
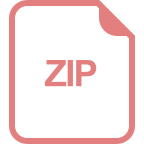
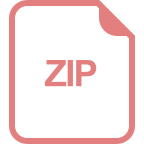
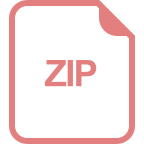

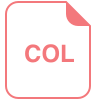

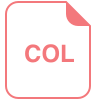
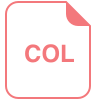









