输入M个班级(每个班级N个学生)学生的计算机文化基础课程成绩,请你设计一个函数来编程找出这些学生中成绩最高的同学(假定最高分只有一名同学),返回最高成绩、该同学是第几个班级的第几个学生(班级和学生编号均从1开始)。
时间: 2024-12-16 19:15:23 浏览: 1
为了设计这样一个函数,我们可以采用以下步骤:
1. 创建一个二维数组或列表来存储每个班级的成绩,其中每个元素表示一个学生在计算机文化基础课程的成绩。例如,可以是一个元组,第一个元素是成绩,第二个和第三个元素分别代表班级ID和学生ID。
```python
class StudentScore:
def __init__(self, score, class_id, student_id):
self.score = score
self.class_id = class_id
self.student_id = student_id
scores = []
```
2. 定义一个辅助函数来比较两个学生的成绩,并返回最高分和对应的索引信息。
```python
def find_highest_score(score1, score2):
if score1[0] > score2[0]:
return score1
else:
return score2
```
3. 主函数遍历整个成绩列表,每次找到当前班级最高分就更新全局的最高分记录。
```python
def find_student_with_highest_score(scores_list):
highest_score = None
best_index = None
for i in range(len(scores_list)):
class_scores = scores_list[i]
for j in range(len(class_scores)):
score = class_scores[j]
if not highest_score or score[0] > highest_score[0]:
highest_score = score
best_index = (i + 1, j + 1) # 班级ID和学生ID加1,因为索引从1开始
return highest_score, best_index
# 示例数据
# scores_list = [
# [85, 1, 1],
# [92, 1, 2],
# [78, 2, 1],
# [98, 2, 2],
# ]
highest_score, top_student_info = find_student_with_highest_score(scores_list)
print("最高成绩:", highest_score[0])
print("学生位置:", "班级", top_student_info[0], ",学生", top_student_info[1])
阅读全文
相关推荐
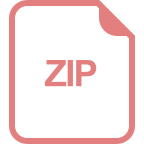
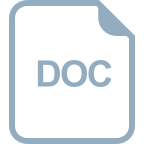
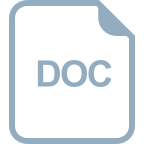

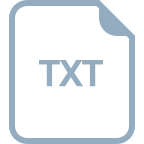
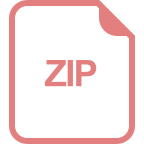
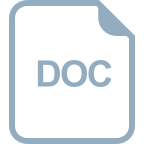
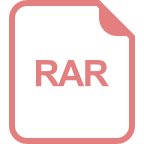
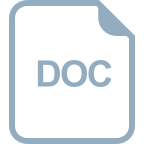
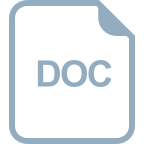
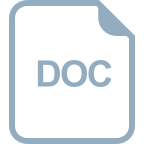
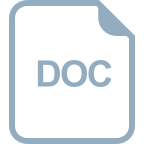
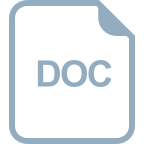
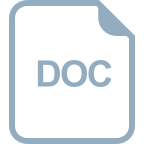
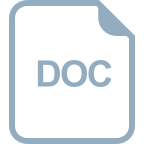
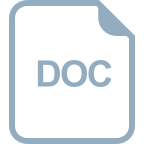
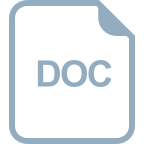

