基于vue学生成绩管理系统
时间: 2024-12-26 10:18:40 浏览: 13
### 使用 Vue.js 开发学生成绩管理系统
#### 项目概述
学生成绩管理系统旨在帮助教育机构管理和记录学生的成绩信息。该系统采用Vue.js作为前端框架,结合Node.js或Spring Boot等后端技术栈来完成数据交互。
#### 技术选型
- **前端**: Vue.js, Axios (HTTP客户端), Element UI 或其他UI库
- **后端**: 可选用Node.js配合Express框架或是Java Spring Boot搭建RESTful API服务[^1]
#### 功能模块划分
为了更好地理解整个应用结构,在此列举几个主要功能点:
- 用户登录/注册页面
- 学生列表展示页
- 成绩录入编辑界面
- 统计分析图表显示区
#### 前端部分实现要点
##### 创建Vue实例并引入Element UI组件库
```javascript
import { createApp } from 'vue';
import App from './App.vue';
// 导入element-ui样式文件以及js脚本
import 'element-plus/lib/theme-chalk/index.css';
import * as El from "element-plus";
const app = createApp(App);
app.use(El); // 注册全局组件
app.mount('#app');
```
##### 定义基础路由配置
通过`vue-router`插件定义不同路径对应加载哪个组件。
```javascript
import {createRouter, createWebHistory} from 'vue-router'
import Login from '@/components/Login.vue'
import StudentList from '@/components/StudentList.vue'
export default createRouter({
history:createWebHistory(),
routes:[
{
path:'/',
name:'login',
component:Login,
},
{
path:'/student-list',
name:'studentlist',
component:StudentList,
meta:{requiresAuth:true}
}
]
})
```
##### 数据获取与渲染
利用Axios发起网络请求向服务器索取所需的数据资源,并将其绑定到模板上呈现给用户查看。
```html
<template>
<div class="container">
<table border="1px solid black" cellpadding="0" cellspacing="0">
<thead><tr><th>ID</th><th>Name</th><th>Score</th></tr></thead>
<tbody v-for="(item,index) in studentsData" :key="index">
<tr>
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.score }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script setup lang='ts'>
import axios from 'axios';
let studentsData=ref([])
onMounted(()=>{
fetchStudents()
})
async function fetchStudents(){
try{
const response=await axios.get('/api/students')
studentsData.value=response.data;
}catch(error){
console.error('Failed to load student data',error)
}
}
</script>
```
阅读全文
相关推荐
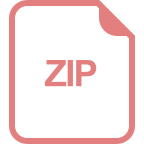
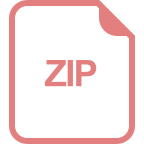
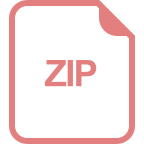
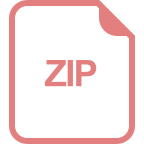
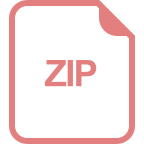
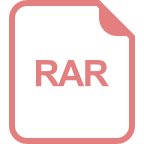
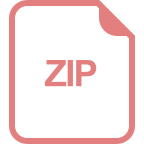
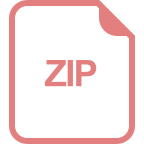
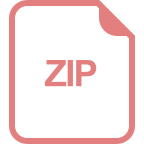
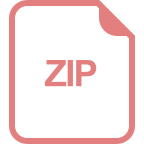
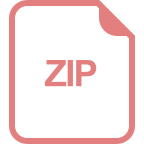
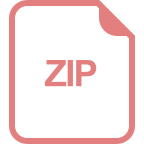
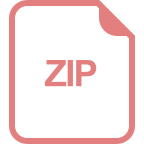
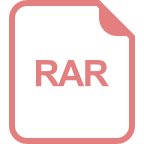
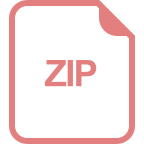
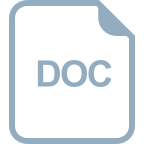
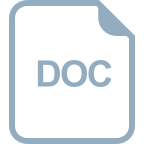