android 通过uuid连接ble 蓝牙
时间: 2023-12-21 17:01:50 浏览: 205
在Android开发中,通过UUID(通用唯一标识符)连接BLE(低功耗蓝牙)蓝牙设备是一种常用的做法。首先,开发人员需要在应用程序中获取并存储目标蓝牙设备的服务UUID和特征UUID。服务UUID通常用于识别设备提供的服务,特征UUID用于读取或写入数据。
接下来,开发人员需要使用Android的BLE API来扫描周围的BLE设备,并过滤出目标设备。一旦找到目标设备,就可以使用其服务UUID和特征UUID来连接设备。
连接BLE设备需要创建一个BluetoothGatt对象,该对象代表了与远程设备的GATT(通用属性配置文件)连接。通过BluetoothDevice对象和BluetoothGattCallback回调接口,开发人员可以请求连接远程设备,发现设备的GATT服务和特征,并读取或写入特征中的数据。
在连接过程中,开发人员可以使用目标设备的服务UUID和特征UUID来识别要读取或写入数据的特征。一旦连接建立并发现了目标设备的服务和特征,就可以通过BluetoothGatt对象进行数据交换,实现与BLE设备的通信。
总的来说,通过UUID连接BLE蓝牙在Android开发中是一个常见的任务,开发人员需要使用Android的BLE API来扫描目标设备,并使用服务UUID和特征UUID来建立连接和进行数据交换。这种技术可以应用于许多领域,如智能穿戴设备、智能家居等。
相关问题
Android BLE蓝牙连接代码
以下是一个简单的 Android BLE 蓝牙连接代码示例:
```
private BluetoothManager bluetoothManager;
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice bluetoothDevice;
private BluetoothGatt bluetoothGatt;
// 初始化 BluetoothManager 和 BluetoothAdapter
bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
bluetoothAdapter = bluetoothManager.getAdapter();
// 扫描设备并连接
bluetoothAdapter.startLeScan(new UUID[]{MY_UUID}, mLeScanCallback);
bluetoothDevice.connectGatt(this, false, mGattCallback);
// 扫描设备的回调函数
private BluetoothAdapter.LeScanCallback mLeScanCallback = new BluetoothAdapter.LeScanCallback() {
@Override
public void onLeScan(final BluetoothDevice device, final int rssi, final byte[] scanRecord) {
if (device.getAddress().equals(DEVICE_ADDRESS)) {
bluetoothDevice = device;
bluetoothAdapter.stopLeScan(mLeScanCallback);
}
}
};
// 连接设备的回调函数
private BluetoothGattCallback mGattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
bluetoothGatt = gatt;
// 连接成功,开始发现服务
bluetoothGatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 连接断开
bluetoothGatt.close();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 发现服务成功,可以开始进行操作
BluetoothGattService service = gatt.getService(SERVICE_UUID);
BluetoothGattCharacteristic characteristic = service.getCharacteristic(CHARACTERISTIC_UUID);
characteristic.setValue("Hello, BLE!");
gatt.writeCharacteristic(characteristic);
}
}
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
// 写入特征值成功
}
};
```
需要注意的是,此示例中的 UUID、DEVICE_ADDRESS、SERVICE_UUID 和 CHARACTERISTIC_UUID 都需要根据实际情况进行替换。
android studio已经连接上了ble蓝牙,如何获取蓝牙发送上来的数据,代码示范
在Android Studio中处理BLE(蓝牙低功耗)设备发送的数据,你需要依赖`BluetoothLeScanner`和`BluetoothGattCallback`。以下是一个简单的步骤和代码示例:
首先,确保你的项目添加了蓝牙权限,并已扫描到设备:
```java
// 扫描蓝牙设备
BluetoothAdapter adapter = BluetoothAdapter.getDefaultAdapter();
if (adapter != null) {
ScanSettings settings = new ScanSettings.Builder()
.setScanMode(ScanSettings.SCAN_MODE_LOW_LATENCY)
.build();
BluetoothLeScanner scanner = adapter.getBluetoothLeScanner();
IntentFilter filter = new IntentFilter();
filter.addAction(BluetoothDevice.ACTION_FOUND);
scanner.startScan(settings, filter, new ScanCallback() {
@Override
public void onScanResult(int callbackType, ScanResult result) {
// 这里可以检查result.getDevice()是否是你感兴趣的设备
if (isValidDevice(result)) {
// 对应的设备ID
String deviceId = result.getDevice().getAddress();
// 使用蓝牙服务获取连接
connectToDevice(deviceId);
}
}
});
}
private boolean isValidDevice(ScanResult result) {
// 根据实际需求判断设备是否满足条件,例如地址、名称等
}
```
然后,在`connectToDevice`方法中连接并获取服务:
```java
private void connectToDevice(String deviceId) {
BluetoothDevice device = BluetoothDevice.connectGatt(this, false, gattCallback);
device.setDiscoverServices(true); // 开始发现服务
}
private BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
gatt.discoverServices(); // 获取所有服务信息
} else {
// 处理断开连接情况
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
for (BluetoothGattService service : gatt.getServices()) {
if (service.getUuid().equals(SERVICE_UUID_YOUR_BLE_SEND_DATA)) { // 替换为你的服务UUID
gatt.getServiceCharacteristics(service.getUuid()); // 获取服务的所有特性
}
}
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
byte[] data = characteristic.getValue();
handleReceivedData(data); // 处理接收到的数据
}
}
};
private void handleReceivedData(byte[] data) {
// 解析data,根据你的数据格式处理这里
// 例如转换成字符串、解析json等
String receivedString = new String(data, StandardCharsets.UTF_8);
// ...然后在这里做你想做的事情,比如更新UI或保存数据
}
```
请注意,这个示例假设你已经有了特定的服务UUID和服务特征UUID。根据实际情况替换相应的值。同时,别忘了在`onCharacteristicRead`回调中处理接收数据的具体逻辑。
阅读全文
相关推荐
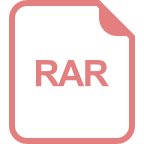
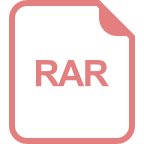
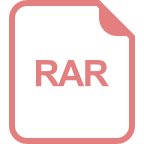
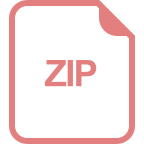
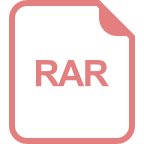
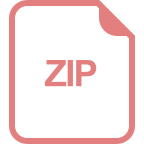
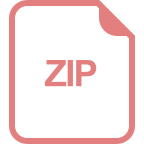
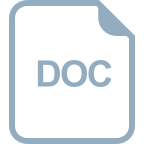
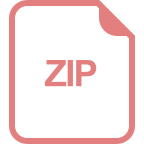
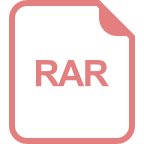
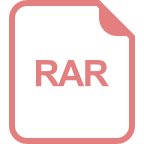
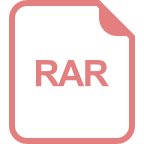
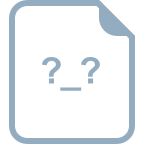
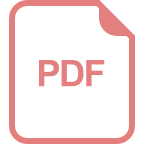