if __name__ == '__main__': array = [] array.append(10) array.append(20) array.pop(0) array.append(30) array.append(40) array.pop(0) print(item)
时间: 2024-04-11 15:28:46 浏览: 39
如果在上述代码中打印 `item`,会引发一个 `NameError` 错误,因为 `item` 没有被定义。如果你想打印 `array` 的值,你可以使用以下代码:
```python
print(array)
```
这将打印出 `[30, 40]`,因为在代码中执行了一系列的 `append` 和 `pop` 操作后,只剩下了 `30` 和 `40` 两个元素。
相关问题
def array_iterate(n: int, input_array: list, m: int) -> list: output_array = [] # 输出数组 choose = 0 # 被选中准备剔除的下标 while input_array: start = choose choose = (start + (m % n) - 1) % n m = input_array.pop(choose) output_array.append(m) n -= 1 return output_array if __name__ == '__main__': input_array = list(input()) lens = int(input()) m = int(input()) res = array_iterate(lens, input_array, m) print(res) 这个代码中的错误如何修改
在这段代码中,存在一个错误。在输入数组 `input_array` 的初始化部分,使用了 `list(input())` 来获取用户输入的列表。然而,`input()` 函数返回的是一个字符串,而不是列表。
要修复这个问题,你可以使用 `split()` 方法将输入的字符串拆分成一个列表。修改后的代码如下所示:
```python
if __name__ == '__main__':
input_array = input().split() # 使用 split() 方法将输入的字符串拆分成列表
lens = int(input())
m = int(input())
res = array_iterate(lens, input_array, m)
print(res)
```
这样,你就可以正确地将用户输入转换为列表,并将其传递给 `array_iterate` 函数进行处理。
if __name__ == "__main__": BMES = [] print("正在读取本地模型矩阵...") with open(r'01/init_mat.pkl', "rb") as f0: init_mat = np.array(list(pickle.load(f0).values())) with open(r'01/trans_mat.pkl', "rb") as f1: init_trans_mat = np.array(list(pickle.load(f1).values())) with open(r'01/emit_mat.pkl', "rb") as f2: init_emit_mat = pickle.load(f2) catalog = list(init_emit_mat) trans_mat = [] emit_mat = [] hidden_state = ["B", "M", "E", "S"] for item in init_trans_mat: trans_mat.append(np.array(list(item.values()))) for i in hidden_state: emit_mat.append(np.array(list(init_emit_mat.loc[i]))) emit_mat = np.array(emit_mat).reshape(4,-1) print("读取模型矩阵成功!") print("目前模型的汉字库",catalog)请给这段代码的每行代码加上详细注释
```
if __name__ == "__main__":
# 定义 BMES 列表
BMES = []
# 打印提示语句
print("正在读取本地模型矩阵...")
# 读取模型中的初始概率矩阵 init_mat
with open(r'01/init_mat.pkl', "rb") as f0:
init_mat = np.array(list(pickle.load(f0).values()))
# 读取模型中的状态转移矩阵 init_trans_mat
with open(r'01/trans_mat.pkl', "rb") as f1:
init_trans_mat = np.array(list(pickle.load(f1).values()))
# 读取模型中的发射概率矩阵 init_emit_mat 和汉字库 catalog
with open(r'01/emit_mat.pkl', "rb") as f2:
init_emit_mat = pickle.load(f2)
catalog = list(init_emit_mat)
# 定义转移矩阵 trans_mat 和发射矩阵 emit_mat
trans_mat = []
emit_mat = []
# 定义隐状态集合
hidden_state = ["B", "M", "E", "S"]
# 将 init_trans_mat 转化为列表形式
for item in init_trans_mat:
trans_mat.append(np.array(list(item.values())))
# 将 init_emit_mat 中的每个状态的发射概率转化为列表形式
for i in hidden_state:
emit_mat.append(np.array(list(init_emit_mat.loc[i])))
# 将发射矩阵 emit_mat 转化为 NumPy 数组形式
emit_mat = np.array(emit_mat).reshape(4,-1)
# 打印提示语句和汉字库
print("读取模型矩阵成功!")
print("目前模型的汉字库",catalog)
```
这段代码的作用是读取本地保存的隐马尔可夫模型(HMM)的参数矩阵,并将其转化为程序中可用的格式。
首先,定义了一个 BMES 列表。接下来,通过打开三个文件,分别读取了初始概率矩阵 init_mat、状态转移矩阵 init_trans_mat 和发射概率矩阵 init_emit_mat,并将这些数据转化为 NumPy 数组或列表形式存储(其中,init_trans_mat 中的每个元素是一个包含状态转移概率的字典)。此外,还提取了 init_emit_mat 中的“汉字库” catalog,即所有可能出现的汉字。
接下来,将 init_trans_mat 转化为列表形式 trans_mat,其中每个元素是一个 NumPy 数组,表示某个状态到其他状态的转移概率。再将 init_emit_mat 中的每个状态的发射概率转化为列表形式 emit_mat,其中每个元素也是一个 NumPy 数组,表示某个状态发射某个汉字的概率。最后,将 emit_mat 转化为 NumPy 数组形式,同时打印出汉字库 catalog 和提示语句。
相关推荐
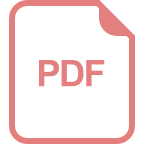
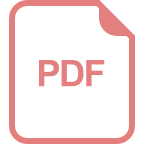
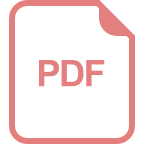












