C语言链表头插入法代码
时间: 2023-12-26 22:26:53 浏览: 37
以下是C语言链表头插入法的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
char data;
struct Node *next;
};
// 头插法创建链表
struct Node *createList() {
struct Node *head = (struct Node *)malloc(sizeof(struct Node));
head->next = NULL;
char c;
while ((c = getchar()) != '\n') {
struct Node *p = (struct Node *)malloc(sizeof(struct Node));
p->data = c;
p->next = head->next;
head->next = p;
}
return head;
}
// 遍历链表
void traverseList(struct Node *head) {
struct Node *p = head->next;
while (p != NULL) {
printf("%c ", p->data);
p = p->next;
}
printf("\n");
}
// 在链表中查找指定元素
struct Node *findNode(struct Node *head, char target) {
struct Node *p = head->next;
while (p != NULL) {
if (p->data == target) {
return p;
}
p = p->next;
}
return NULL;
}
// 在链表中删除指定元素
void deleteNode(struct Node *head, char target) {
struct Node *p = head->next;
struct Node *pre = head;
while (p != NULL) {
if (p->data == target) {
pre->next = p->next;
free(p);
return;
}
pre = p;
p = p->next;
}
}
// 单向链表反转
struct Node *reverseList(struct Node *head) {
struct Node *pre = NULL;
struct Node *cur = head->next;
while (cur != NULL) {
struct Node *next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
}
head->next = pre;
return head;
}
int main() {
// 创建链表
struct Node *head = createList();
// 遍历链表
traverseList(head);
// 查找元素
char target = 'a';
struct Node *node = findNode(head, target);
if (node != NULL) {
printf("Found node with data %c\n", target);
} else {
printf("Node with data %c not found\n", target);
}
// 删除元素
target = 'b';
deleteNode(head, target);
printf("After deleting node with data %c: ", target);
traverseList(head);
// 反转链表
head = reverseList(head);
printf("After reversing the list: ");
traverseList(head);
return 0;
}
```
相关推荐
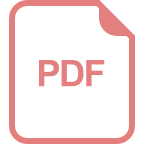
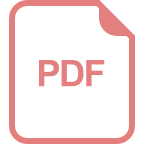
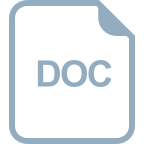














