c语言 在有序链表中插入数据
时间: 2024-03-14 11:16:18 浏览: 104
在有序链表中插入数据需要遵循以下步骤:
1. 创建一个新节点,将待插入数据存储在新节点中。
2. 遍历链表,找到插入位置。从链表头开始依次比较每个节点的值,直到找到第一个大于待插入值的节点。
3. 将新节点插入到链表中。如果插入位置是链表头,则将新节点作为新的头节点,否则将新节点插入到比它大的节点之前。
下面是一个示例代码:
```
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head_ref, int new_data)
{
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
struct Node* current = *head_ref;
struct Node* prev = NULL;
while (current != NULL && current->data < new_data) {
prev = current;
current = current->next;
}
if (prev == NULL) {
new_node->next = *head_ref;
*head_ref = new_node;
} else {
new_node->next = current;
prev->next = new_node;
}
}
```
在这个示例代码中,我们遍历链表找到插入位置,然后将新节点插入到链表中。如果插入位置是链表头,则将新节点作为新的头节点,否则将新节点插入到比它大的节点之前。请注意,此代码假设链表已按升序排列。
阅读全文
相关推荐
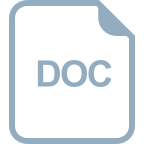
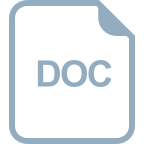
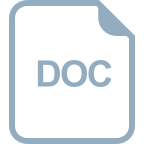




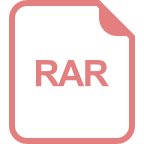
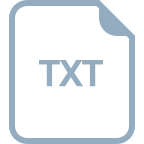
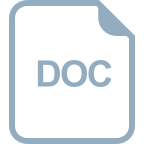
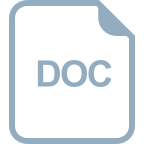
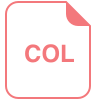






