用c语言完成:链表中元素已经按从小到大有序排放,编程将用户输入的数据元素插入到表中适当位置,并保持链表的有序性。
时间: 2023-05-11 21:02:14 浏览: 94
可以使用以下代码实现:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
struct Node* current = *head_ref;
struct Node* prev = NULL;
while (current != NULL && current->data < new_data) {
prev = current;
current = current->next;
}
if (prev == NULL) {
new_node->next = *head_ref;
*head_ref = new_node;
} else {
prev->next = new_node;
new_node->next = current;
}
}
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct Node* head = NULL;
insert(&head, 1);
insert(&head, 3);
insert(&head, 5);
insert(&head, 7);
printf("Original list: ");
printList(head);
int new_data;
printf("\nEnter new data to be inserted: ");
scanf("%d", &new_data);
insert(&head, new_data);
printf("Updated list: ");
printList(head);
return 0;
}
```
这个程序会创建一个链表,然后按照从小到大的顺序插入一些数据。然后,它会要求用户输入一个新的数据元素,并将其插入到链表中适当的位置,以保持链表的有序性。最后,它会输出更新后的链表。
相关推荐
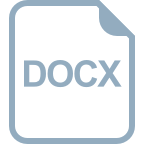
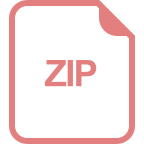














