编写程序,从键盘输入十个数,将这些整数插入到一个带头结点双向链表中,并从小到大排列
时间: 2024-10-06 12:01:13 浏览: 38
编写程序来实现这个功能,你需要首先创建一个双向链表的数据结构,包含头节点和两个指针(前驱和后继)。然后按照以下步骤操作:
1. **定义数据结构**:
- 创建一个`Node`类,用于表示链表中的每个元素,包含`value`(数值)、`prev`(前驱节点引用) 和 `next`(后继节点引用)。
2. **获取用户输入**:
- 使用`cin`循环读取用户的10个整数输入。
3. **初始化双向链表**:
- 创建头节点`head`,设置`prev`和`next`指向自身,`value`设为第一个输入的数字。
4. **遍历并插入**:
- 对于剩余的9个输入,依次创建新节点并将它们插入到已排序的部分,保证链表始终有序。对于每一个新节点,比较其值与当前头节点的值,如果小于当前值,则将其插入到头节点之前;否则,遍历到合适的位置插入。
5. **排序算法**:
- 可以选择简单的插入排序方法,每次找到适当位置插入,直到所有元素插入完毕。
6. **打印链表**:
- 最后,遍历整个链表,通过`next`指针输出链表内容,确保按顺序显示。
```cpp
#include <iostream>
using namespace std;
struct Node {
int value;
Node* prev;
Node* next;
};
void insert(Node*& head, int num) {
if (head == nullptr || head->value > num) {
Node* newNode = new Node{num, nullptr, head};
if (head != nullptr) {
head->prev = newNode;
}
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr && current->next->value < num) {
current = current->next;
}
Node* newNode = new Node{num, current, current->next};
current->next->prev = newNode;
current->next = newNode;
}
}
void printList(Node* head) {
Node* temp = head;
while (temp != nullptr) {
cout << temp->value << " ";
temp = temp->next;
}
cout << endl;
}
int main() {
Node* head = nullptr;
for (int i = 0; i < 10; ++i) {
int num;
cin >> num;
insert(head, num);
}
printList(head);
return 0;
}
```
阅读全文
相关推荐
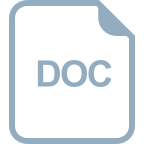
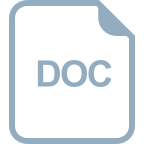
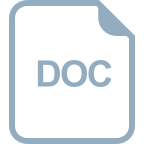








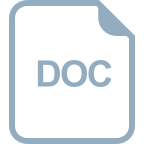







