c语言版在有序链表中插入数据
时间: 2024-03-14 15:17:05 浏览: 19
以下是C语言版在有序链表中插入数据的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
// 在有序链表中插入数据
Node *insert(Node *head, int value) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
// 如果链表为空,直接插入
if (head == NULL) {
head = newNode;
return head;
}
// 如果插入的值小于等于头节点的值,插入到头节点前面
if (value <= head->data) {
newNode->next = head;
head = newNode;
return head;
}
// 否则在链表中查找插入位置
Node *current = head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
return head;
}
// 打印链表
void printList(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 测试代码
int main() {
Node *head = NULL;
head = insert(head, 1);
head = insert(head, 3);
head = insert(head, 2);
head = insert(head, 5);
head = insert(head, 4);
printList(head);
return 0;
}
```
输出结果为:1 2 3 4 5
相关推荐
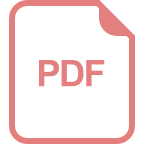
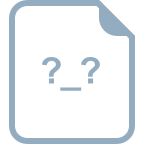














