帮我写一个简单的在有序链表中插入数据的简单程序用c++
时间: 2024-04-30 07:17:08 浏览: 17
好的,下面是一个简单的在有序链表中插入数据的C语言程序:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int val;
struct Node* next;
};
// 在链表中插入一个节点,节点的值为val
struct Node* insert(struct Node* head, int val) {
// 创建新节点
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->val = val;
new_node->next = NULL;
// 如果链表为空或插入的节点值比head节点大,则插入到链表头部
if (head == NULL || val < head->val) {
new_node->next = head;
return new_node;
}
// 否则,找到插入节点的位置并插入
struct Node* curr = head;
while (curr->next != NULL && curr->next->val < val) {
curr = curr->next;
}
new_node->next = curr->next;
curr->next = new_node;
return head;
}
int main() {
struct Node* head = NULL;
// 初始化链表
for (int i = 1; i <= 5; i++) {
head = insert(head, i);
}
// 插入新节点
head = insert(head, 3);
// 输出链表
struct Node* curr = head;
while (curr != NULL) {
printf("%d ", curr->val);
curr = curr->next;
}
return 0;
}
```
该程序中的insert函数实现了在有序链表中插入一个节点的功能。首先,创建一个新节点,然后判断链表是否为空或插入的节点值比head节点大。如果是,则将新节点插入到链表的头部;否则,遍历链表找到插入节点的位置并插入。
在main函数中,首先初始化一个有序链表,然后将一个新节点插入到链表中,并输出整个链表。运行程序,输出结果如下:
```
1 2 3 3 4 5
```
可以看到,在链表中成功地插入了一个值为3的节点。
相关推荐
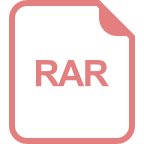














