帮我写一个在有序链表中插入数据的c++程序
时间: 2024-05-01 15:19:27 浏览: 108
好的,以下是一个简单的在有序链表中插入数据的 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* next;
} Node;
void insert(Node** head, int data) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = data;
new_node->next = NULL;
if (*head == NULL || (*head)->data >= data) {
new_node->next = *head;
*head = new_node;
}
else {
Node* current = *head;
while (current->next != NULL && current->next->data < data) {
current = current->next;
}
new_node->next = current->next;
current->next = new_node;
}
}
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
insert(&head, 3);
insert(&head, 1);
insert(&head, 5);
insert(&head, 2);
printList(head);
return 0;
}
```
这个程序首先定义了一个结构体用于表示链表中的节点,其中包括一个整数数据和一个指向下一个节点的指针。在 `insert` 函数中,我们创建一个新节点,并将其插入到链表中正确的位置。在这个程序中,链表是按升序排列的,每次插入数据都会寻找正确的位置并插入到那里。最后,我们使用 `printList` 函数来打印链表中的所有节点。
阅读全文
相关推荐








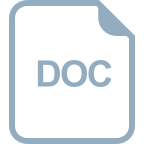






