帮我写一个简单的在严格递增的有序链表中插入数据的简单程序用c++
时间: 2024-05-14 12:15:52 浏览: 21
好的,下面是一个简单的在严格递增的有序链表中插入数据的程序:
```
#include<stdio.h>
#include<stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* head;
void insert(int x) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode -> data = x;
newNode -> next = NULL;
if(head == NULL || x < head -> data) {
newNode -> next = head;
head = newNode;
return;
}
struct Node* current = head;
while(current -> next != NULL && current -> next -> data < x) {
current = current -> next;
}
newNode -> next = current -> next;
current -> next = newNode;
}
void printList() {
struct Node* current = head;
while(current != NULL) {
printf("%d ", current -> data);
current = current -> next;
}
printf("\n");
}
int main() {
head = NULL;
insert(10);
insert(20);
insert(30);
insert(40);
printf("List after insertion: ");
printList();
return 0;
}
```
在这个程序中,我们使用了一个 `struct` 来定义节点 `Node`,其中包括一个整数 `data` 和一个指向下一个节点的指针 `next`。我们还定义了一个头节点 `head`,初始时为 `NULL`,表示链表为空。
`insert` 函数用于在链表中插入一个新的元素。我们首先创建一个新的节点 `newNode`,将其 `data` 设置为要插入的值 `x`,并将其 `next` 设置为 `NULL`。然后,我们检查链表是否为空或者要插入的值 `x` 是否小于头节点的值,如果是,则将 `newNode` 插入到头节点前,并修改头节点 `head` 的指向。否则,我们遍历链表,找到 `x` 要插入的位置,并将 `newNode` 插入其中。
`printList` 函数用于遍历整个链表,并输出每个节点的元素值。
在 `main` 函数中,我们初始化头节点 `head` 为空,然后插入若干个元素并打印整个链表。
相关推荐
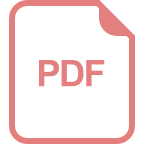
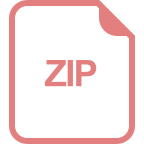
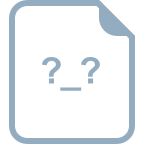
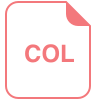
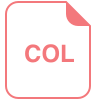
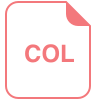
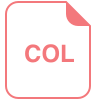
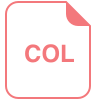









