用c++完成完成单链表中的元素实现有序递增
时间: 2023-05-28 10:05:28 浏览: 152
以下是用C语言实现单链表中元素有序递增的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 在有序单链表中插入新节点
struct ListNode* insert(struct ListNode *head, int val) {
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = NULL;
if (head == NULL) {
head = node;
} else if (val < head->val) {
node->next = head;
head = node;
} else {
struct ListNode *cur = head;
while (cur->next != NULL && val >= cur->next->val) {
cur = cur->next;
}
node->next = cur->next;
cur->next = node;
}
return head;
}
// 打印单链表
void printList(struct ListNode *head) {
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
// 主函数
int main() {
struct ListNode *head = NULL;
head = insert(head, 3);
head = insert(head, 1);
head = insert(head, 5);
head = insert(head, 2);
head = insert(head, 4);
printList(head);
return 0;
}
```
在上述代码中,我们定义了一个单链表的节点结构体 ListNode,其中包含节点的值 val 和指向下一个节点的指针 next。我们还定义了 insert 函数,用于在有序单链表中插入新节点,并保持单链表有序递增。在插入节点时,我们首先判断单链表是否为空,如果为空,则将新节点作为头节点;如果新节点的值小于头节点的值,则将新节点插入到头节点之前;否则,我们遍历单链表,找到新节点应该插入的位置,并将其插入到链表中。最后,我们定义了 printList 函数,用于打印单链表中的所有节点值。在主函数中,我们测试了 insert 函数的正确性,并通过 printList 函数打印了单链表中的所有节点值。
阅读全文
相关推荐
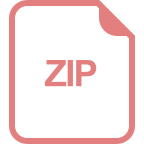
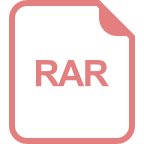
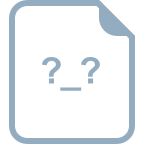















