帮我写一个在有序链表中插入数据的简单的c++代码
时间: 2024-05-08 15:18:29 浏览: 102
好的,以下是一个简单的在有序链表中插入数据的 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insertSorted(struct Node** headRef, int newData) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->next = NULL;
if (*headRef == NULL || (*headRef)->data >= newNode->data) {
newNode->next = *headRef;
*headRef = newNode;
} else {
struct Node* current = *headRef;
while (current->next != NULL && current->next->data < newNode->data) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct Node* head = NULL;
insertSorted(&head, 5);
insertSorted(&head, 10);
insertSorted(&head, 7);
insertSorted(&head, 3);
insertSorted(&head, 1);
printf("Sorted LinkedList: ");
printList(head);
return 0;
}
```
在这个代码中,我们定义了一个结构体 `Node`,它包括一个数据元素和一个指向下一个节点的指针。`insertSorted` 函数用于将新元素插入到已排好序的链表中,其中 `headRef` 是作为一个指向指针的指针传递给函数的,因为要更改链表的头指针。
`printList` 函数用于遍历链表并将其打印到控制台上。在 `main` 函数中,我们创建了一个链表并将它们按顺序插入到链表中,然后打印出排序后的链表。
阅读全文
相关推荐
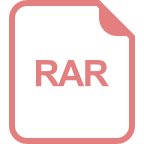




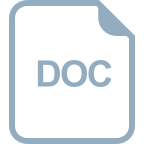
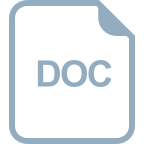









