c语言创建有序链表
时间: 2023-12-03 08:04:20 浏览: 42
好的,以下是用C语言创建一个有序链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode* next;
};
// 插入元素到有序链表中
struct ListNode* insert(struct ListNode* head, int val) {
// 创建新节点
struct ListNode* new_node = (struct ListNode*)malloc(sizeof(struct ListNode));
new_node->val = val;
new_node->next = NULL;
if (head == NULL) {
// 如果链表为空,则直接插入新节点
head = new_node;
} else if (head->val > val) {
// 如果新节点的值小于链表头结点的值,则将新节点作为新的头结点
new_node->next = head;
head = new_node;
} else {
// 遍历链表,找到合适的插入位置
struct ListNode* cur = head;
while (cur->next && cur->next->val < val) {
cur = cur->next;
}
new_node->next = cur->next;
cur->next = new_node;
}
return head;
}
// 打印链表
void print_list(struct ListNode* head) {
struct ListNode* cur = head;
while (cur) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
struct ListNode* head = NULL;
// 插入元素到有序链表中
head = insert(head, 5);
head = insert(head, 3);
head = insert(head, 7);
head = insert(head, 1);
head = insert(head, 9);
// 打印有序链表
print_list(head);
return 0;
}
```
这个示例代码中,我们定义了一个链表节点结构体 `ListNode`,包含了一个 `val` 域和一个指向下一个节点的指针 `next`。然后实现了一个 `insert` 函数,用于将一个新的元素插入到有序链表中。当链表为空时,直接插入新节点;当新节点的值小于链表头结点的值时,将新节点作为新的头结点;否则,遍历链表找到合适的插入位置。最后,我们用 `print_list` 函数打印出整个有序链表。
相关推荐
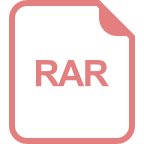
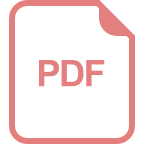
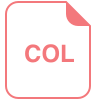
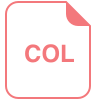
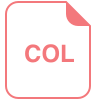
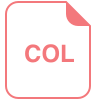
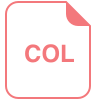









