字符串数字提取c++代码
时间: 2023-08-16 11:09:20 浏览: 214
当你说提取字符串中的数字,我理解为从字符串中提取出数字部分。这里是一个简单的C++代码示例,可以帮助你实现这个功能:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "abc123def456";
std::string numStr = "";
for (char c : str) {
if (std::isdigit(c)) {
numStr += c;
}
}
std::cout << "提取到的数字为:" << numStr << std::endl;
return 0;
}
```
以上代码将提取字符串 `str` 中的所有数字,并将它们存储在字符串 `numStr` 中。在循环中,我们使用 `std::isdigit()` 函数来检查字符是否为数字,并将数字字符添加到 `numStr` 中。
运行代码后,输出将是:
```
提取到的数字为:123456
```
你可以根据自己的需求修改代码,适应不同的字符串提取数字的场景。
相关问题
c++从字符串中提取数字求和
可以使用正则表达式来从字符串中提取数字,然后将这些数字相加。具体代码如下:
```cpp
#include <iostream>
#include <regex>
#include <string>
using namespace std;
int main()
{
string str = "abc123def456ghi789";
regex reg("\\d+");
smatch match;
int sum = 0;
while (regex_search(str, match, reg)) {
for (auto x : match) {
sum += stoi(x);
}
str = match.suffix().str();
}
cout << "Sum of all numbers in the string: " << sum << endl;
return 0;
}
```
以上代码会输出:Sum of all numbers in the string: 1368,这是因为字符串中的数字分别为123、456和789,它们的和为1368。
用c++在字符串里提取数字
可以使用C语言提供的字符处理函数,例如isdigit()、atoi()等来实现字符串中数字的提取。
一个简单的实现示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[] = "abc123def456";
int num1 = 0, num2 = 0;
int i = 0;
while (str[i]) {
if (isdigit(str[i])) {
num1 = num2;
num2 = atoi(&str[i]);
printf("Found number: %d\n", num2);
i += 1;
while (isdigit(str[i])) i += 1;
} else {
i += 1;
}
}
return 0;
}
```
这个示例代码会在字符串中寻找数字,并将数字提取出来打印出来。如果需要获取所有数字,可以将 num1 和 num2 保存在一个数组中。
阅读全文
相关推荐
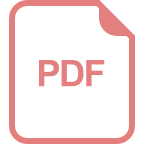
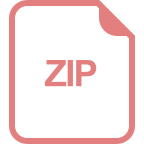
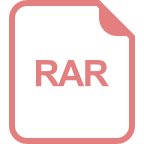
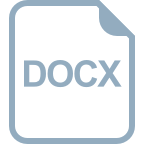
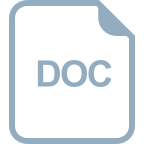
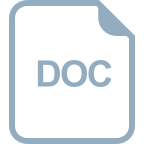









