springboot实现分页查询mongodb
时间: 2024-10-28 13:10:30 浏览: 29
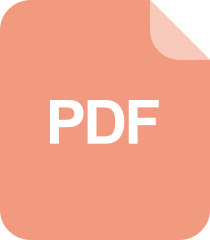
springboot+mongodb 实现按日期分组分页查询功能
Spring Boot结合MongoDB进行分页查询通常涉及以下几个步骤:
1. 添加依赖:首先,在你的Maven或Gradle项目中添加Spring Data MongoDB的依赖,以及Spring Web的依赖,因为分页需要HTTP支持。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb-reactive</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
// Gradle
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb-reactive'
implementation 'org.springframework.boot:spring-boot-starter-webflux'
```
2. 配置MongoTemplate:在`application.yml`或`application.properties`文件中配置MongoDB连接信息。
```yaml
spring:
data:
mongodb:
host: localhost
port: 27017
database: your-database-name
```
3. 使用MongoRepository:Spring Data MongoDB提供了一个名为`MongoRepository`的接口,它已经包含了基本的CRUD操作,包括查询方法。你需要创建一个泛型接口,比如`YourEntityRepository`,其中包含`Pageable`类型的查询方法。
```java
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.data.mongodb.core.query.Pageable;
public interface YourEntityRepository extends MongoRepository<YourEntity, String> {
Page<YourEntity> findAll(Pageable pageable);
}
```
4. 在服务层或控制器中调用:通过注入你的repository实例,然后传入`PageRequest`对象来指定分页参数,如页码、每页大小等。
```java
@Autowired
private YourEntityRepository repository;
@GetMapping("/api/v1/entities")
public Flux pageableEntities(@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Pageable pageable = PageRequest.of(page, size);
return repository.findAll(pageable);
}
```
阅读全文
相关推荐
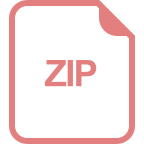
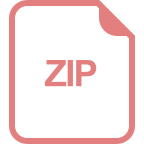
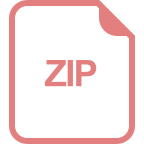
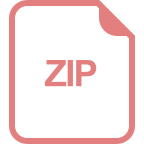
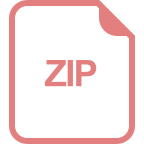
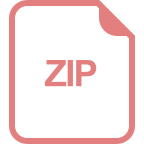
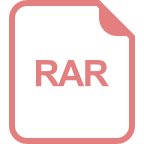
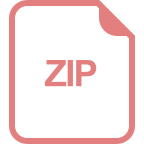
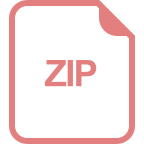
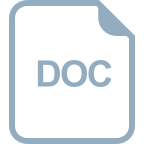
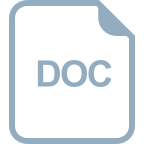

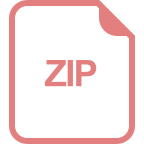

