用Python写一个银行密码管理系统
时间: 2023-02-24 17:48:54 浏览: 508
为了写一个银行密码管理系统,您可以使用 Python。 您可以使用 Python 内置的数据结构,如字典或列表,来存储用户的账户信息,包括用户名和密码。 然后,您可以编写一些代码来提供密码验证功能,并在验证通过后允许用户进行各种操作,例如查询余额,转账或更改密码。
以下是一些可以帮助您开始的代码示例:
```
# 银行密码管理系统
# 存储用户信息的字典
user_info = {
"user1": "password1",
"user2": "password2",
"user3": "password3"
}
# 用户登录
def login():
username = input("请输入用户名:")
password = input("请输入密码:")
if username in user_info and user_info[username] == password:
print("登录成功!")
else:
print("用户名或密码错误,请重试。")
# 启动程序
if __name__ == "__main__":
login()
```
请注意,这仅是一个简单的示例,没有考虑到安全性和可靠性,因此请勿在生产环境中使用此代码。
相关问题
用python写一个银行管理系统
抱歉,作为AI语言模型,我不能编写完整的程序。但我可以提供一些基本的功能和代码结构,您可以根据这些内容进行编写和完善。
基本功能:
1. 用户注册和登录:包括输入用户名、密码等信息,验证用户身份;
2. 存款和取款:用户可以输入存款或取款金额,系统会更新用户账户余额;
3. 转账:用户可以输入转账金额和转账目标账户,系统会进行转账操作并更新账户余额;
4. 查询余额:用户可以查询自己的账户余额;
5. 修改密码:用户可以修改自己的密码。
代码结构:
1. 定义用户类:包括用户的基本信息、账户余额等属性,以及存款、取款、转账、查询余额等方法;
2. 定义银行类:包括所有用户信息的存储、用户注册和登录、修改密码等方法;
3. 主程序:提供用户界面和交互功能,包括用户输入、调用相关方法等。
例如,以下是一个简单的银行管理系统的代码示例:
```python
class User:
def __init__(self, name, password, balance):
self.name = name
self.password = password
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient balance")
else:
self.balance -= amount
def transfer(self, amount, target):
if amount > self.balance:
print("Insufficient balance")
else:
self.balance -= amount
target.deposit(amount)
def check_balance(self):
print("Your balance is:", self.balance)
def change_password(self, new_password):
self.password = new_password
class Bank:
def __init__(self):
self.users = {}
def register(self):
name = input("Enter your name: ")
password = input("Enter your password: ")
balance = int(input("Enter your initial balance: "))
user = User(name, password, balance)
self.users[name] = user
print("Registration successful")
def login(self):
name = input("Enter your name: ")
password = input("Enter your password: ")
if name in self.users and self.users[name].password == password:
print("Login successful")
return self.users[name]
else:
print("Invalid name or password")
def change_password(self):
user = self.login()
if user:
new_password = input("Enter your new password: ")
user.change_password(new_password)
print("Password changed successfully")
def main_menu(self):
print("1. Register")
print("2. Login")
print("3. Change password")
print("4. Exit")
choice = int(input("Enter your choice: "))
if choice == 1:
self.register()
elif choice == 2:
user = self.login()
if user:
self.user_menu(user)
elif choice == 3:
self.change_password()
elif choice == 4:
exit()
def user_menu(self, user):
print("1. Deposit")
print("2. Withdraw")
print("3. Transfer")
print("4. Check balance")
print("5. Logout")
choice = int(input("Enter your choice: "))
if choice == 1:
amount = int(input("Enter the deposit amount: "))
user.deposit(amount)
print("Deposit successful")
self.user_menu(user)
elif choice == 2:
amount = int(input("Enter the withdrawal amount: "))
user.withdraw(amount)
print("Withdrawal successful")
self.user_menu(user)
elif choice == 3:
target_name = input("Enter the target user name: ")
amount = int(input("Enter the transfer amount: "))
if target_name in self.users:
target = self.users[target_name]
user.transfer(amount, target)
print("Transfer successful")
else:
print("Invalid target user")
self.user_menu(user)
elif choice == 4:
user.check_balance()
self.user_menu(user)
elif choice == 5:
self.main_menu()
bank = Bank()
while True:
bank.main_menu()
```
注意:这只是一个简单的示例代码,可能存在一些风险,如未对用户输入进行验证等。实际应用中,需要更加严格地处理用户输入和操作。
帮我写一份python的银行管理系统 要求拥有更改密码功能
在Python中编写一个简单的银行管理系统,我们可以创建一个BankAccount类,它包含基本的操作如存款、取款和更改密码的功能。下面是一个基础的实现示例:
```python
class BankAccount:
def __init__(self, account_number, balance, password):
self.account_number = account_number
self.balance = balance
self.password = password
def change_password(self, old_password, new_password):
if self.verify_password(old_password):
self.password = new_password
print(f"Password successfully changed to: {new_password}")
else:
print("Incorrect password. Change failed.")
def verify_password(self, password):
# 这里只是一个简化的验证,实际应用中应更安全地存储密码
return password == "your_initial_password"
def deposit(self, amount):
if amount > 0:
self.balance += amount
print(f"Deposited {amount}, new balance: {self.balance}")
else:
print("Invalid deposit amount.")
def withdraw(self, amount):
if amount <= self.balance:
self.balance -= amount
print(f"Withdrew {amount}, new balance: {self.balance}")
else:
print("Insufficient balance for withdrawal.")
# 示例用户操作
account = BankAccount("123456", 1000, "your_initial_password")
account.change_password("your_initial_password", "new_password")
account.deposit(500)
account.withdraw(200)
```
在这个例子中,用户需要输入初始密码来创建账户并更改密码。请注意,这只是一个非常基础的实现,真实的银行系统会更复杂,包括对用户身份的验证、数据持久化以及安全性更高的密码处理。
阅读全文
相关推荐
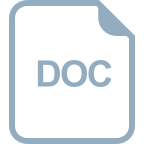
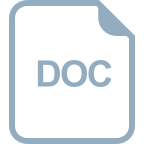
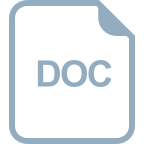
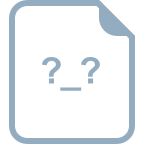
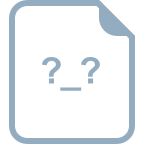
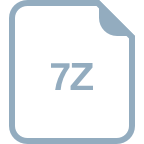
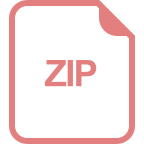
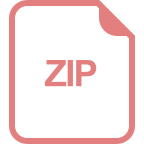



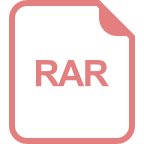
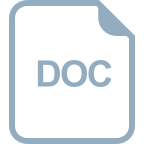


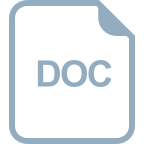