Python银行管理系统简易代码
时间: 2024-03-28 14:31:52 浏览: 109
以下是一个Python版简易银行管理系统的代码示例,实现了开户、账户查询、存取款、转账、改密、锁定、解锁、补卡、销户等银行管理操作。账户信息存在allusers.txt中,通过该文件的读存来实现账户的管理。
```python
import os
# 定义用户类
class User:
def __init__(self, name, password, balance=0):
self.name = name
self.password = password
self.balance = balance
self.is_locked = False
# 存款
def deposit(self, amount):
self.balance += amount
print("存款成功,当前余额为:", self.balance)
# 取款
def withdraw(self, amount):
if amount > self.balance:
print("余额不足,取款失败")
else:
self.balance -= amount
print("取款成功,当前余额为:", self.balance)
# 转账
def transfer(self, to_user, amount):
if self.balance < amount:
print("余额不足,转账失败")
else:
self.balance -= amount
to_user.balance += amount
print("转账成功,当前余额为:", self.balance)
# 修改密码
def change_password(self, new_password):
self.password = new_password
print("密码修改成功")
# 锁定账户
def lock(self):
self.is_locked = True
print("账户已锁定")
# 解锁账户
def unlock(self):
self.is_locked = False
print("账户已解锁")
# 补卡
def reissue_card(self):
print("补卡成功")
# 销户
def close_account(self):
print("账户已销户")
# 定义银行类
class Bank:
def __init__(self):
self.users = {}
self.load_users()
# 加载用户信息
def load_users(self):
if not os.path.exists("allusers.txt"):
return
with open("allusers.txt", "r") as f:
for line in f:
name, password, balance, is_locked = line.strip().split(",")
self.users[name] = User(name, password, int(balance))
if is_locked == "True":
self.users[name].is_locked = True
# 保存用户信息
def save_users(self):
with open("allusers.txt", "w") as f:
for user in self.users.values():
f.write("{},{},{},{}\n".format(user.name, user.password, user.balance, user.is_locked))
# 开户
def create_account(self, name, password):
if name in self.users:
print("该用户已存在")
else:
self.users[name] = User(name, password)
self.save_users()
print("开户成功")
# 登录
def login(self, name, password):
if name not in self.users:
print("该用户不存在")
elif self.users[name].is_locked:
print("该账户已被锁定,请联系管理员")
elif self.users[name].password != password:
print("密码错误")
else:
print("登录成功")
# 查询余额
def check_balance(self, name):
if name not in self.users:
print("该用户不存在")
else:
print("当前余额为:", self.users[name].balance)
# 修改密码
def change_password(self, name, old_password, new_password):
if name not in self.users:
print("该用户不存在")
elif self.users[name].is_locked:
print("该账户已被锁定,请联系管理员")
elif self.users[name].password != old_password:
print("原密码错误")
else:
self.users[name].change_password(new_password)
self.save_users()
# 锁定账户
def lock_account(self, name):
if name not in self.users:
print("该用户不存在")
else:
self.users[name].lock()
self.save_users()
# 解锁账户
def unlock_account(self, name):
if name not in self.users:
print("该用户不存在")
else:
self.users[name].unlock()
self.save_users()
# 补卡
def reissue_card(self, name):
if name not in self.users:
print("该用户不存在")
else:
self.users[name].reissue_card()
self.save_users()
# 销户
def close_account(self, name):
if name not in self.users:
print("该用户不存在")
else:
self.users.pop(name)
self.save_users()
print("账户已销户")
# 示例代码
bank = Bank()
bank.create_account("张三", "123456")
bank.login("张三", "123456")
bank.check_balance("张三")
bank.deposit("张三", 1000)
bank.check_balance("张三")
bank.withdraw("张三", 500)
bank.check_balance("张三")
bank.create_account("李四", "123456")
bank.transfer("张三", "李四", 200)
bank.check_balance("张三")
bank.check_balance("李四")
bank.change_password("张三", "123456", "654321")
bank.lock_account("张三")
bank.login("张三", "123456")
bank.unlock_account("张三")
bank.login("张三", "123456")
bank.reissue_card("张三")
bank.close_account("张三")
```
阅读全文
相关推荐









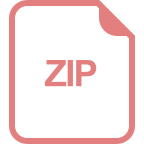







