#include<stdio.h> #include<stdlib.h> struct Node{ int data; struct Node* next; }; struct Node* head; void Insert(int data){ struct Node* temp1 = (struct Node*)malloc(sizeof(struct Node*));//创建一个新的节点 temp1->data = data; temp1->next = NULL; struct Node* temp2 = head; if (temp2==NULL){ //头节点为NULL // temp1->next = temp2; temp2 = temp1; temp1->next = NULL; return; } while(temp2->next!=NULL){ //temp2->next!=NULL即第一个节点的链接位置不为空 //就指向下一个位置 temp2=temp2->next; // return; } //即temp2指向temp1 temp2->next = temp1; }; void Print(){ struct Node* temp = head; while(temp!=NULL){ printf("%d ",temp->data); temp=temp->next; } printf("\n"); }; void Delete(int n); int main(){ head = NULL; Insert(2); Insert(4); Insert(6); Insert(5);//2 4 5 6 Print(); // int n; // printf("enter a position\n"); // scanf("%d",&n); // Delete(n); // Print(); }这段代码有什么问题
时间: 2024-01-25 12:03:07 浏览: 159
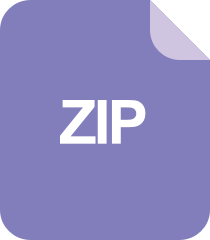
SeqList.h #include <stdio.h> //包含标准输入输出流的头文件 #include <assert.h
这段代码存在以下问题:
1. 在 `struct Node* temp1 = (struct Node*)malloc(sizeof(struct Node*));` 中,应该将 `sizeof(struct Node*)` 改为 `sizeof(struct Node)`。`malloc` 函数需要传入需要分配内存的字节数,而 `sizeof` 运算符返回的是类型的大小,所以应该使用 `sizeof(struct Node)`。
2. 在 `Insert` 函数中,当头节点为 `NULL` 时,插入新节点的逻辑有问题。将节点链接的语句应该写在 `temp1` 插入到链表中之后,而不是之前。应该修改为:
```c
if (temp2 == NULL) {
head = temp1; // 头节点为NULL时,将头节点指向temp1
return;
}
```
3. 在 `Delete` 函数中,未定义该函数的实现。如果要使用该函数,需要先定义该函数的实现。
4. 在 `main` 函数中,没有调用 `Delete` 函数。如果要测试 `Delete` 函数的实现,需要调用该函数。
阅读全文
相关推荐
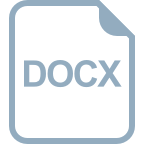
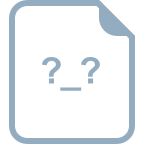
















