时间轴都是第一列,请重写代码
时间: 2023-12-31 20:04:01 浏览: 25
好的,如果两个表格的时间轴都是第一列,那么你可以按照以下步骤实现:
1. 找到两个表格中的所有时间轴数据。
2. 对两个表格中的时间轴数据进行去重,得到一个包含所有时间轴数据的列表。
3. 针对每个时间轴数据,分别在两个表格中查找对应的数据行。
4. 如果两个表格中都存在对应的数据行,则将这两行数据合并到一个新的表格中。
5. 如果只存在一个表格中,则将这行数据复制到新表格中。
6. 将所有合并后的数据行组成一个新的表格返回。
你可以使用 Python 中的 Pandas 库来实现这个过程。具体实现细节可以参考以下代码示例:
```python
import pandas as pd
# 读取两个表格
df1 = pd.read_excel('table1.xlsx')
df2 = pd.read_excel('table2.xlsx')
# 取出两个表格中的时间轴数据并去重
all_times = pd.concat([df1.iloc[:, 0], df2.iloc[:, 0]]).unique()
# 创建一个新表格
new_table = pd.DataFrame(columns=df1.columns)
# 遍历所有时间轴数据
for time in all_times:
# 在两个表格中查找对应的数据行
rows1 = df1[df1.iloc[:, 0] == time]
rows2 = df2[df2.iloc[:, 0] == time]
# 如果两个表格中都存在对应的数据行,则将这两行数据合并到新表格中
if not rows1.empty and not rows2.empty:
new_row = pd.concat([rows1, rows2.iloc[:, 1:]], axis=1)
new_table = new_table.append(new_row, ignore_index=True)
# 如果只存在一个表格中,则将这行数据复制到新表格中
elif not rows1.empty:
new_table = new_table.append(rows1, ignore_index=True)
elif not rows2.empty:
new_table = new_table.append(rows2, ignore_index=True)
# 输出新表格
print(new_table)
```
其中,`table1.xlsx` 和 `table2.xlsx` 分别是两个表格的文件名,需要替换成你实际使用的文件名。在输出新表格时,你可以将 `print(new_table)` 替换成其他操作,比如将新表格保存到文件中。
相关推荐
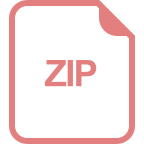
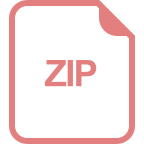
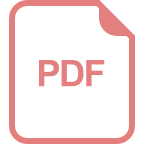














