int main() { char command = '\0'; while (command != 'g') { menu(); scanf("%c", &command); while (command < 'a' || command > 'z') { scanf("%c", &command); } switch (command) //选择需要的功能 { case 'a': inputScores(); //成绩录入 continue; case 'b': displayScores(); //成绩显示 continue; case 'c': saveScores(); //成绩保存 continue; case 'd': orderScores(); //成绩排序 continue; case 'e': changeScores(); //成绩修改 continue; case 'f': statisticScores(); //成绩统计 continue; case 'g': //退出系统 break; default: printf("Unknown choice!\n"); continue; } } return 0; }
时间: 2024-02-10 20:20:21 浏览: 8
这段代码是一个简单的成绩管理系统,通过命令行菜单实现不同的功能,包括成绩录入、成绩显示、成绩保存、成绩排序、成绩修改和成绩统计。其中,输入命令的字符必须为小写字母,否则会提示输入错误。当命令为'g'时,程序退出。这段代码使用了while循环和switch语句来实现菜单的功能选择,同时使用了函数来实现具体的操作。
相关问题
int main() { char command = '\0'; while (command != 'g') { menu(); scanf("%c", &command); while (command < 'a' || command > 'z') { scanf("%c", &command); } switch (command) //选择需要的功能 { case 'a': inputScores(); //成绩录入 continue; case 'b': displayScores(); //成绩显示 continue; case 'c': saveScores(); //成绩保存 continue; case 'd': orderScores(); //成绩排序 continue; case 'e': changeScores(); //成绩修改 continue; case 'f': statisticScores(); //成绩统计 continue; case 'g': //退出系统 break; default: printf("Unknown choice!\n"); continue; } } return 0; }
这段代码是一个简单的成绩管理系统,它包含一个while循环,每次迭代都会显示一个菜单,接收用户输入的命令,并根据命令执行相应的操作。在每次输入命令后,使用while循环检查输入的命令是否合法,如果不合法则重新接收命令。如果命令为 'g' 则退出程序。该程序可以录入成绩、显示成绩、保存成绩、排序成绩、修改成绩和统计成绩等功能。
C语言排错#include <stdio.h> #include <stdlib.h> unsigned int NumofChar(char * str,char ch) { int count=0; char *p=str; while(*p!='\0') { if((*p++)==ch) {count++;} } return count; } char * DelChar(char * str,char ch,unsigned int n) { char *s=str; int co=0; while(co<n) { if(*s==ch) { co++; for(char* p=s;*p!='\0';p++) *p=*(p+1); s--; } s++; }a return s; } int main() { char str[100]; printf("Please input the string!\n"); gets(str); char c; printf("Please input the character!\n"); scanf("%c",&c); unsigned int count=NumofChar(str,c); if(count==0) { printf("Not found!"); return 0; } printf("The number of %c is %d!\nWhich one do you want to delete?Please input the serial number:\n",c,count); int sn;//第几个数字 scanf("%d",&sn); printf("%s",DelChar(str,c,sn)); return 0; }
在函数 `DelChar` 的第 21 行,多了一个 `a` 字符,需要删除。此外,在删除字符的过程中,应该先把 `s` 指针移动到要删除的字符位置,然后再进行删除操作。修改后的代码如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
unsigned int NumofChar(char * str,char ch) {
int count=0;
char *p=str;
while(*p!='\0')
{
if((*p++)==ch)
{count++;}
}
return count;
}
char * DelChar(char * str,char ch,unsigned int n) {
char *s=str;
int co=0;
while(co<n && *s!='\0')
{
if(*s==ch)
{
co++;
char* p=s;
while (*p!='\0')
{
*p=*(p+1);
p++;
}
s--;
}
s++;
}
return str;
}
int main() {
char str[100];
printf("Please input the string!\n");
gets(str);
char c;
printf("Please input the character!\n");
scanf("%c",&c);
unsigned int count=NumofChar(str,c);
if(count==0)
{
printf("Not found!");
return 0;
}
printf("The number of %c is %d!\nWhich one do you want to delete?Please input the serial number:\n",c,count);
int sn;//第几个数字
scanf("%d",&sn);
printf("%s",DelChar(str,c,sn));
return 0;
}
```
阅读全文
相关推荐
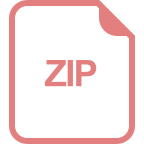
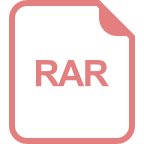











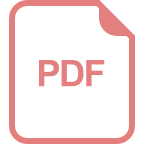
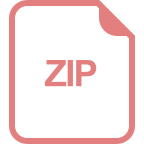
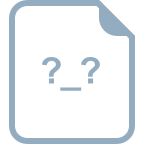