解读一下这段代码#define MAX_INPUT_LENGTH 100 #define MAX_DATA_COUNT 10 int main() { char input[MAX_INPUT_LENGTH + 1]; fgets(input, MAX_INPUT_LENGTH + 1, stdin); input[strcspn(input, "\n")] = '\0'; // 去掉末尾的换行符 char *p = input; char *datas[MAX_DATA_COUNT]; int dataslen = 0; while ((p = strtok(p, ","))) { datas[dataslen++] = p; p = NULL; } for (int i = 0; i < dataslen - 1; i++) { for (int j = 0; j < dataslen - 1 - i; j++) { char tmp1[MAX_INPUT_LENGTH * 2 + 1]; char tmp2[MAX_INPUT_LENGTH * 2 + 1]; sprintf(tmp1, "%s%s", datas[j], datas[j + 1]); sprintf(tmp2, "%s%s", datas[j + 1], datas[j]); if (strcmp(tmp1, tmp2) < 0) { char *tmp = datas[j]; datas[j] = datas[j + 1]; datas[j + 1] = tmp; } } } for (int i = 0; i < dataslen; i++) { printf("%s", datas[i]); } return 0; }
时间: 2023-09-26 19:08:58 浏览: 175
这段代码实现了一个字符串排序的功能,具体实现步骤如下:
1. 定义了两个常量 MAX_INPUT_LENGTH 和 MAX_DATA_COUNT,分别表示输入字符串的最大长度和最大数据数量。
2. 从标准输入中读取一行字符串,存储到字符数组 input 中。如果输入的字符串长度大于 MAX_INPUT_LENGTH,则只读取前 MAX_INPUT_LENGTH 个字符。
3. 使用 strcspn 函数去掉字符串末尾的换行符。
4. 定义指向 input 的指针 p,以及一个字符指针数组 datas,用于存储被逗号分隔后的字符串片段。
5. 使用 strtok 函数对 input 进行分割,将每个逗号分隔的字符串片段存储到 datas 数组中。
6. 使用冒泡排序算法对 datas 数组进行排序,排序的依据是将相邻的两个字符串连接后进行比较,若前者小于后者,则交换它们的位置。
7. 输出排序后的字符串数组。
需要注意的是,在排序算法中使用了 sprintf 函数将两个字符串连接起来,为了确保拼接后字符串的长度不会超过 MAX_INPUT_LENGTH * 2,定义了两个临时字符数组 tmp1 和 tmp2 来存储拼接后的字符串。
相关问题
#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_SIZE 100 typedef struct { int book_id; char book_name[50]; float price; } Book; typedef struct { Book books[MAX_SIZE]; int length; } BookList; void input_books(BookList* list, int n) { for (int i = 0; i < n; i++) { printf("请输入第%d本书的信息:\n", i + 1); printf("图书编号:"); scanf("%d", &list->books[i].book_id); printf("书名:"); scanf("%s", list->books[i].book_name); printf("价格:"); scanf("%f", &list->books[i].price); } list->length = n; } void display_books(BookList* list) { printf("图书表中所有图书的相关信息:\n"); for (int i = 0; i < list->length; i++) { printf("图书编号:%d\n", list->books[i].book_id); printf("书名:%s\n", list->books[i].book_name); printf("价格:%f\n", list->books[i].price); } } void insert_book(BookList* list, int pos, Book book) { if (pos < 1 || pos > list->length + 1) { printf("插入位置不合法!\n"); return; } for (int i = list->length - 1; i >= pos - 1; i--) { list->books[i + 1] = list->books[i]; } list->books[pos - 1] = book; list->length++; } void delete_book(BookList* list, int pos) { if (pos < 1 || pos > list->length) { printf("删除位置不合法!\n"); return; } for (int i = pos - 1; i < list->length - 1; i++) { list->books[i] = list->books[i + 1]; } list->length--; } int count_books(BookList* list) { return list->length; } int partition(BookList* list, int low, int high) { Book pivot = list->books[low]; while (low < high) { while (low < high && list->books[high].book_id >= pivot.book_id) high--; list->books[low] = list->books[high]; while (low < high && list->books[low].book_id <= pivot.book_id) low++; list->books[high] = list->books[low]; } list->books[low] = pivot; return low; } void quick_sort(BookList* list, int
) {
if (list->length <= 1) return;
int stack[MAX_SIZE], top = -1;
stack[++top] = 0;
stack[++top] = list->length - 1;
while (top > 0) {
int high = stack[top--];
int low = stack[top--];
int mid = partition(list, low, high);
if (low < mid - 1) {
stack[++top] = low;
stack[++top] = mid - 1;
}
if (mid + 1 < high) {
stack[++top] = mid + 1;
stack[++top] = high;
}
}
}
int main() {
BookList list;
input_books(&list, 3);
display_books(&list);
Book book = { 4, "Data Structures and Algorithms", 45.8 };
insert_book(&list, 2, book);
display_books(&list);
delete_book(&list, 3);
display_books(&list);
int count = count_books(&list);
printf("图书表中共有%d本图书。\n", count);
quick_sort(&list, count);
display_books(&list);
return 0;
}
这是一个图书管理系统的代码,实现了图书的增删查改和快速排序功能。其中Book和BookList为自定义的结构体,分别表示图书和图书表。在主函数中,先输入3本图书的信息,然后显示所有图书的信息,接着在第二个位置插入一本新书并显示所有图书的信息,再删除第三本书并显示所有图书的信息。然后统计图书数量并输出,最后对图书表进行快速排序并输出排序结果。
修改此代码使其效率变高int main(){ char input[100]={0}; gets(input); char *p = input; char datas[10][10]={0}; int dataslen = 0; while(p= strtok(p,",")){ strcpy(datas[dataslen],p); dataslen++; p=NULL; } for(int i =0;i<dataslen-1;i++){ for(int j =0;j<dataslen-1-i;j++){ char tmp1[100]={0}; char tmp2[100]={0}; sprintf(tmp1,"%s%s",datas[j],datas[j+1]); sprintf(tmp2,"%s%s",datas[j+1],datas[j]); if(strcmp(tmp1,tmp2)<0){ strcpy(input,datas[j]); strcpy(datas[j],datas[j+1]); strcpy(datas[j+1],input); } } } for(int i =0;i<dataslen;i++){ printf("%s",datas[i]); } }
可以使用更高效的输入函数替代 `gets()`,比如 `fgets()`。同时,可以使用指针数组代替二维字符数组,避免不必要的内存分配和复制操作。此外,在排序时可以使用更高效的排序算法,如快速排序。
修改后的代码如下:
```c++
#include <stdio.h>
#include <string.h>
#define MAX_INPUT_LENGTH 100
#define MAX_DATA_COUNT 10
int main() {
char input[MAX_INPUT_LENGTH + 1];
fgets(input, MAX_INPUT_LENGTH + 1, stdin);
input[strcspn(input, "\n")] = '\0'; // 去掉末尾的换行符
char *p = input;
char *datas[MAX_DATA_COUNT];
int dataslen = 0;
while ((p = strtok(p, ","))) {
datas[dataslen++] = p;
p = NULL;
}
for (int i = 0; i < dataslen - 1; i++) {
for (int j = 0; j < dataslen - 1 - i; j++) {
char tmp1[MAX_INPUT_LENGTH * 2 + 1];
char tmp2[MAX_INPUT_LENGTH * 2 + 1];
sprintf(tmp1, "%s%s", datas[j], datas[j + 1]);
sprintf(tmp2, "%s%s", datas[j + 1], datas[j]);
if (strcmp(tmp1, tmp2) < 0) {
char *tmp = datas[j];
datas[j] = datas[j + 1];
datas[j + 1] = tmp;
}
}
}
for (int i = 0; i < dataslen; i++) {
printf("%s", datas[i]);
}
return 0;
}
```
这个修改后的代码使用了指针数组 `datas` 存储输入中的字符串,并在排序时直接交换指针,避免了大量的内存分配和复制操作。同时,使用了更高效的输入函数 `fgets()`,并去掉了 `gets()` 容易引发的缓冲区溢出问题。
阅读全文
相关推荐
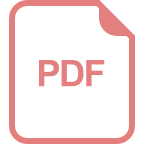
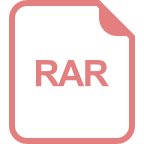
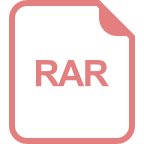









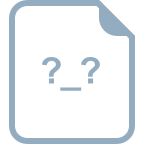
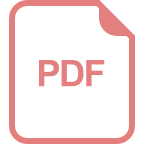
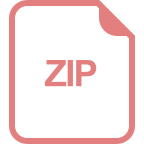
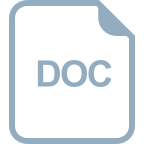
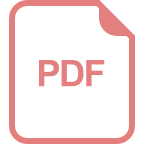