android app调用系统相机后拍照,将照片路径信息存到数据库,并在recyclerview显示拍到的照片
时间: 2023-12-17 18:05:35 浏览: 29
实现这个功能需要以下几个步骤:
1. 在 AndroidManifest.xml 文件中添加相机和文件读写权限。
```
<uses-permission android:name="android.permission.CAMERA"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
```
2. 创建一个拍照按钮,并在点击事件中调用系统相机。
```java
// 启动系统相机
public void startCamera() {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (intent.resolveActivity(getPackageManager()) != null) {
File photoFile = createImageFile();
if (photoFile != null) {
Uri photoUri = FileProvider.getUriForFile(this, "com.example.fileprovider", photoFile);
intent.putExtra(MediaStore.EXTRA_OUTPUT, photoUri);
startActivityForResult(intent, REQUEST_CODE_CAMERA);
}
}
}
// 创建图片文件
public File createImageFile() {
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
try {
File imageFile = File.createTempFile(imageFileName, ".jpg", storageDir);
mCurrentPhotoPath = imageFile.getAbsolutePath();
return imageFile;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
// 处理拍照结果
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_CODE_CAMERA && resultCode == RESULT_OK) {
String photoPath = mCurrentPhotoPath;
savePhotoPath(photoPath); // 将照片路径保存到数据库
mAdapter.addData(photoPath); // 将照片路径添加到 RecyclerView 中显示
}
}
```
3. 在 Activity 中创建一个 RecyclerView,并通过 Adapter 将数据绑定到 RecyclerView 上。
```java
// 初始化 RecyclerView
public void initRecyclerView() {
mRecyclerView = findViewById(R.id.recyclerview);
mRecyclerView.setLayoutManager(new LinearLayoutManager(this));
mAdapter = new PhotoAdapter(this);
mRecyclerView.setAdapter(mAdapter);
}
// 创建 Adapter
public class PhotoAdapter extends RecyclerView.Adapter<PhotoAdapter.ViewHolder> {
private Context mContext;
private List<String> mPhotoPaths;
public PhotoAdapter(Context context) {
mContext = context;
mPhotoPaths = new ArrayList<>();
}
public void setData(List<String> photoPaths) {
mPhotoPaths.clear();
mPhotoPaths.addAll(photoPaths);
notifyDataSetChanged();
}
public void addData(String photoPath) {
mPhotoPaths.add(0, photoPath);
notifyItemInserted(0);
mRecyclerView.scrollToPosition(0);
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(mContext).inflate(R.layout.item_photo, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
String photoPath = mPhotoPaths.get(position);
Glide.with(mContext).load(photoPath).into(holder.ivPhoto);
}
@Override
public int getItemCount() {
return mPhotoPaths.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
ImageView ivPhoto;
public ViewHolder(View itemView) {
super(itemView);
ivPhoto = itemView.findViewById(R.id.iv_photo);
}
}
}
```
4. 将拍摄的照片路径保存到数据库中。
```java
// 将照片路径保存到数据库
public void savePhotoPath(String photoPath) {
SQLiteDatabase db = mDbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(PhotoEntry.COLUMN_NAME_PATH, photoPath);
db.insert(PhotoEntry.TABLE_NAME, null, values);
db.close();
}
```
5. 在 Activity 的 onCreate() 方法中初始化 RecyclerView 和数据库。
```java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initRecyclerView();
mDbHelper = new PhotoDbHelper(this);
loadPhotoPaths(); // 从数据库中加载照片路径并显示到 RecyclerView 上
}
```
6. 从数据库中加载照片路径并显示到 RecyclerView 上。
```java
// 从数据库中加载照片路径并显示到 RecyclerView 上
public void loadPhotoPaths() {
SQLiteDatabase db = mDbHelper.getReadableDatabase();
String[] projection = {
PhotoEntry._ID,
PhotoEntry.COLUMN_NAME_PATH
};
Cursor cursor = db.query(
PhotoEntry.TABLE_NAME,
projection,
null,
null,
null,
null,
PhotoEntry._ID + " DESC"
);
List<String> photoPaths = new ArrayList<>();
while (cursor.moveToNext()) {
String photoPath = cursor.getString(cursor.getColumnIndexOrThrow(PhotoEntry.COLUMN_NAME_PATH));
photoPaths.add(photoPath);
}
mAdapter.setData(photoPaths);
cursor.close();
db.close();
}
```
这样就可以实现在 Android App 中调用系统相机后拍照,将照片路径信息存到数据库,并在 RecyclerView 显示拍到的照片。
相关推荐
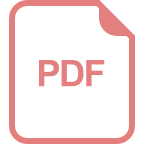
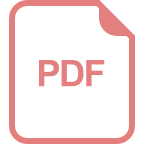














