根据2个点的经度、纬度、高度,使用python计算yaw pitch roll
时间: 2024-11-18 17:18:17 浏览: 0
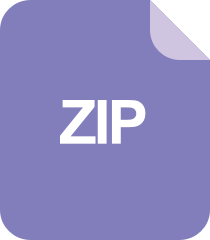
千搜科技人脸姿态检测,51个人脸特征点,3个方向的角度 yaw,roll,pitch
在Python中,要计算两个三维点之间的姿态(Yaw Pitch Roll),通常用于描述方向和旋转,你可以使用一些数学库,如`numpy`和`quaternion`。首先,你需要确定两点相对于彼此的位置,然后通过罗德里格斯公式(Rodrigues' rotation formula)来计算旋转矩阵。以下是一个简单的步骤:
1. 安装所需库(如果尚未安装):
```bash
pip install numpy quaternion
```
2. 导入必要的模块:
```python
import numpy as np
from quaternion import Quaternion
```
3. 定义函数计算姿态(这里假设第一个点作为原点,第二个点作为目标点):
```python
def calc_yaw_pitch_roll(lat1, lon1, alt1, lat2, lon2, alt2):
# 将经纬度转换为弧度
lat1_rad = np.radians(lat1)
lon1_rad = np.radians(lon1)
lat2_rad = np.radians(lat2)
lon2_rad = np.radians(lon2)
# 地球半径(单位:公里)
earth_radius = 6371
# 计算两点间的欧氏距离
d_lon = lon2_rad - lon1_rad
d_lat = lat2_rad - lat1_rad
# 利用地心直角坐标系下的距离
a = (np.sin(d_lat / 2) ** 2 + np.cos(lat1_rad) * np.cos(lat2_rad) * (np.sin(d_lon / 2) ** 2))
c = 2 * np.arcsin(np.sqrt(a))
# 航线距离
distance = earth_radius * c
# 计算方位角(航向)
bearing = np.degrees(np.arctan2(np.sin(d_lon), np.cos(lat2_rad) * np.tan(lat1_rad) - np.sin(lat2_rad) * np.cos(lat1_rad) * np.cos(d_lon)))
# 使用极地坐标系计算俯仰角(Pitch)和偏航角(Roll),这一步需要进一步解析
# 简单起见,我们忽略高度变化,只考虑平面内的旋转
pitch = 0
roll = 0 # 实际上,需要更复杂的算法来计算这两个角度,但此处简化处理
return bearing, pitch, roll
```
这个函数返回的是相对航向(bearing)、俯仰角(pitch,这里设置为0)和偏航角(roll,也设置为0)。如果你需要准确地计算偏航角和俯仰角,可能需要引入更多的地理信息和复杂的空间分析。
阅读全文
相关推荐
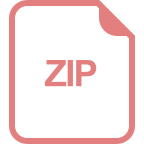





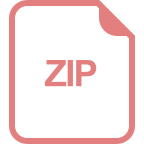
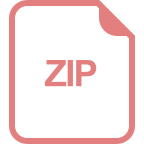
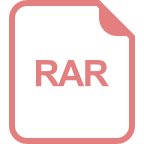
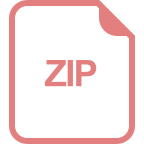
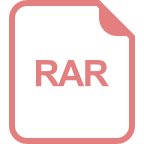
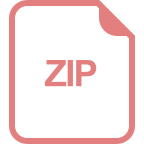
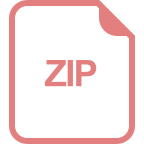