怎么让bluedroid协议栈AVDTP连接前不跳过SDP查询
时间: 2024-01-07 13:02:55 浏览: 22
在Bluedroid协议栈中,可以通过修改配置文件来控制AVDTP连接前不能跳过SDP查询。具体的做法如下:
1. 打开Bluedroid协议栈的配置文件。在Android源代码中,该文件位于 `system/bt/bdroid_buildcfg.h`。
2. 在配置文件中添加以下代码:
```c
#define AVDT_CFG_NON_DISC FALSE
```
这行代码的作用是禁用AVDTP连接前的非发现模式。在非发现模式下,AVDTP连接可以直接使用存储的SDP记录,而不进行实时的SDP查询。
3. 保存配置文件,并重新编译Bluedroid协议栈。
这样,在AVDTP连接建立前,就不会跳过SDP查询步骤,从而保证了连接的稳定性和可靠性。但需要注意的是,这种方式可能会导致连接速度变慢,因为每次建立连接都需要进行实时的SDP查询。
相关问题
怎么让bluedroid建立AVDTP前发起SDP服务查询
在Android系统中,可以通过Bluedroid协议栈提供的API接口来建立AVDTP前发起SDP服务查询。具体步骤如下:
1. 打开蓝牙,并检查是否支持AVDTP协议。
2. 创建一个SDP查询,用于查询指定UUID的服务。
3. 将查询请求发送到远程设备,并等待响应。
4. 解析响应,获取远程设备支持的服务列表。
5. 如果远程设备支持AVDTP协议,则可以通过AVDTP API接口来建立AVDTP连接。
下面是具体的代码实现:
```
BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice remoteDevice = mBluetoothAdapter.getRemoteDevice(deviceAddress);
// 检查是否支持AVDTP协议
if (remoteDevice.getBluetoothClass().hasService(BluetoothClass.Service.AUDIO)) {
// 创建一个SDP查询
UUID uuid = BluetoothUuid.AudioSource;
String serviceName = "";
int protocolType = Protocol.SDP;
ParcelUuid[] uuids = new ParcelUuid[] { new ParcelUuid(uuid) };
int[] attributeIds = new int[] { Attribute.SERVICE_NAME };
BluetoothSdpSearchParam searchParam = new BluetoothSdpSearchParam(uuids, attributeIds);
BluetoothSdpRecord record = null;
BluetoothSdpService sdpService = null;
BluetoothSdpServiceClass serviceClass = null;
BluetoothSdpServiceName serviceNameObj = null;
BluetoothSdpServiceAttribute serviceNameAttr = null;
// 发送查询请求并等待响应
BluetoothSdp sdp = new BluetoothSdp(context, null, null);
List<BluetoothDevice> deviceList = new ArrayList<BluetoothDevice>();
deviceList.add(remoteDevice);
sdp.searchServices(deviceList, searchParam);
List<BluetoothSdpRecord> recordList = sdp.getRecords();
if (recordList != null && recordList.size() > 0) {
record = recordList.get(0);
sdpService = record.getService();
serviceClass = sdpService.getServiceClass();
serviceNameAttr = sdpService.getAttribute(Attribute.SERVICE_NAME);
if (serviceNameAttr != null) {
serviceNameObj = (BluetoothSdpServiceName) serviceNameAttr.getValue();
serviceName = serviceNameObj.toString();
}
}
// 解析响应,获取远程设备支持的服务列表
if (serviceClass != null && serviceClass.hasService(BluetoothUuid.AudioSource)) {
// 远程设备支持AVDTP协议,可以建立AVDTP连接
BluetoothAvrcpController avrcpController = new BluetoothAvrcpController(context, null, null);
BluetoothAvrcpDevice avrcpDevice = avrcpController.getDevice(remoteDevice);
avrcpDevice.connect();
}
}
```
以上代码通过Bluedroid协议栈提供的BluetoothSdp API接口来发起SDP服务查询,并解析响应结果。如果远程设备支持AVDTP协议,则可以建立AVDTP连接。
怎么让bluedroid协议栈在发起AVDTP的l2cap通道建立前,先发起sdp audio sink/source查询。
在Android中,BlueDroid是蓝牙协议栈的实现,它是基于BlueZ的开源项目。要在BlueDroid协议栈中发起SDP(Service Discovery Protocol)查询,可以通过实现BluetoothProfile.ServiceListener来监听SDP操作是否完成,然后再进行后续的操作。
以下是大致的步骤:
1. 首先,需要创建一个BluetoothAdapter对象,用于获取本地蓝牙适配器。
```
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
```
2. 然后,需要创建一个BluetoothDevice对象,用于表示远程蓝牙设备。
```
BluetoothDevice bluetoothDevice = bluetoothAdapter.getRemoteDevice(deviceAddress);
```
3. 接着,需要创建一个BluetoothA2dpSink或BluetoothA2dp源对象,用于表示A2DP Sink或A2DP源。
```
BluetoothA2dpSink bluetoothA2dpSink = new BluetoothA2dpSink(context, serviceListener);
```
或者
```
BluetoothA2dp bluetoothA2dp = new BluetoothA2dp(context, serviceListener);
```
4. 然后,需要在BluetoothProfile.ServiceListener中实现onServiceConnected方法,这个方法会在SDP查询完成后被调用。在该方法中,你可以根据查询结果进行后续的操作,例如建立L2CAP通道。
```
BluetoothProfile.ServiceListener serviceListener = new BluetoothProfile.ServiceListener() {
@Override
public void onServiceConnected(int profile, BluetoothProfile proxy) {
if (profile == BluetoothProfile.A2DP_SINK) {
BluetoothA2dpSink bluetoothA2dpSink = (BluetoothA2dpSink) proxy;
// SDP查询完成后的操作
} else if (profile == BluetoothProfile.A2DP) {
BluetoothA2dp bluetoothA2dp = (BluetoothA2dp) proxy;
// SDP查询完成后的操作
}
}
@Override
public void onServiceDisconnected(int profile) {
// Service disconnected
}
};
```
可以在onServiceConnected方法中先发起SDP查询,查询完成后再发起L2CAP通道建立。
需要注意的是,具体的实现方法会因具体情况而异,具体的实现细节需要根据具体情况进行调整。
相关推荐
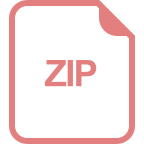
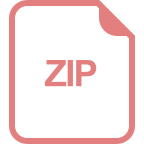
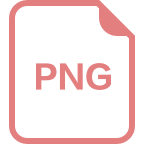










