python进行边界处理,将插值点投影到最近的三角形边界上是一种常见的处理方法。
时间: 2024-04-15 17:28:26 浏览: 118
在Python中,可以使用以下代码来实现将插值点投影到最近的三角形边界上的边界处理方法:
```python
import numpy as np
def project_to_boundary(triangle, point):
vertices = triangle[:2] # 提取三角形的前两个顶点坐标
edges = np.vstack((vertices, vertices[0])) # 构造三条边的起点和终点坐标
# 计算插值点到三条边的垂直距离
distances = np.abs(np.cross(edges[1:] - edges[:-1], edges[:-1] - point)) / np.linalg.norm(edges[1:] - edges[:-1], axis=1)
# 找到离插值点最近的边
nearest_edge_index = np.argmin(distances)
nearest_edge = edges[nearest_edge_index:nearest_edge_index + 2]
# 计算插值点在最近边上的投影点
t = np.dot(point - nearest_edge[0], nearest_edge[1] - nearest_edge[0]) / np.dot(nearest_edge[1] - nearest_edge[0], nearest_edge[1] - nearest_edge[0])
projected_point = nearest_edge[0] + t * (nearest_edge[1] - nearest_edge[0])
return projected_point
# 示例使用
triangle = np.array([[0, 0], [1, 0], [0, 1]]) # 三角形的顶点坐标
point = np.array([0.5, -0.5]) # 插值点的坐标
projected_point = project_to_boundary(triangle, point)
print(projected_point)
```
上述代码中,`project_to_boundary` 函数接受三角形的顶点坐标和插值点坐标作为参数,返回投影到最近边界上的点的坐标。其中,使用向量操作和线性代数计算实现了向量的投影计算。
在示例中,三角形的顶点坐标为 `[[0, 0], [1, 0], [0, 1]]`,插值点的坐标为 `[0.5, -0.5]`。运行代码后,将得到投影点的坐标。
请注意,上述代码仅提供了一种基本的实现方法,具体的应用场景和需求可能需要进行适当的修改和优化。
阅读全文
相关推荐
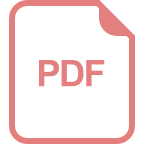
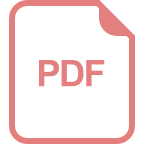
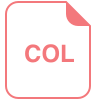
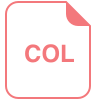
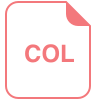
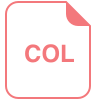
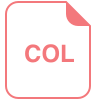
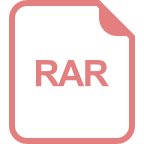
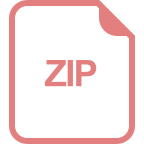
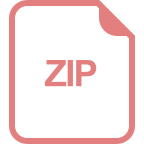
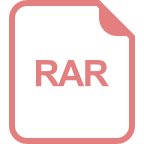
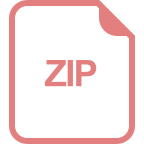
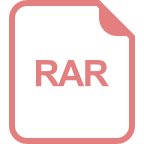
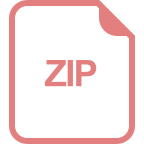
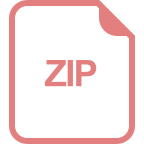