揭秘GIS中的Delaunay三角剖分:地理信息处理的利器
发布时间: 2024-07-07 20:40:12 阅读量: 132 订阅数: 41 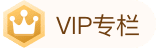
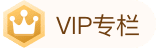
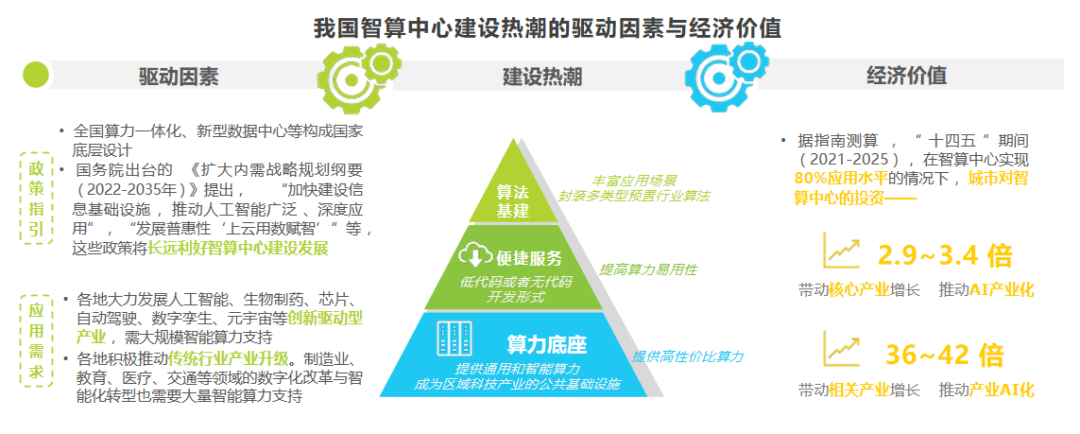
# 1. Delaunay三角剖分概述
Delaunay三角剖分是一种空间数据结构,它将一组点集划分为一系列不重叠的三角形。这些三角形具有以下性质:
- 每个三角形的圆心都不包含其他点。
- 每个三角形的边都是其相邻三角形的公共边。
Delaunay三角剖分在计算机图形学、地理信息系统和计算几何等领域有着广泛的应用。它可以用于地形建模、空间插值、路径规划和网络分析等任务。
# 2. Delaunay三角剖分的理论基础
### 2.1 Voronoi图与Delaunay三角剖分的联系
Voronoi图和Delaunay三角剖分是密切相关的几何结构。Voronoi图将平面划分为一系列多边形,每个多边形对应于一个生成点。对于任何生成点,其Voronoi多边形包含了所有离该点比其他任何生成点更近的所有点。
Delaunay三角剖分是Voronoi图的的对偶结构。对于每个Voronoi多边形,都有一个对应的Delaunay三角形,其顶点是该多边形的生成点。反之亦然,对于每个Delaunay三角形,都有一个对应的Voronoi多边形,其边缘是该三角形的边。
### 2.2 Delaunay三角剖分的数学模型
Delaunay三角剖分可以形式化为一个数学优化问题。目标是找到一组三角形,使得每个三角形都是Delaunay三角形,即对于任何三角形,都不存在一个点在该三角形内或其边界上,并且离该三角形的三个顶点都比其他任何生成点更近。
这个优化问题可以通过以下线性规划模型来表示:
```
最小化:∑∑(x_i - x_j)^2 + (y_i - y_j)^2
约束条件:
x_i, y_i >= 0, ∀i
x_i - x_j + y_i - y_j <= 0, ∀i, j, i ≠ j
```
其中,(x_i, y_i)表示生成点i的坐标。
这个模型的目标函数表示要最小化的三角形边长的平方和。约束条件确保生成的三角形是Delaunay三角形,因为它们保证了对于任何三角形,都不存在一个点在该三角形内或其边界上,并且离该三角形的三个顶点都比其他任何生成点更近。
通过求解这个线性规划模型,可以得到Delaunay三角剖分。
# 3. Delaunay三角剖分的算法实现
### 3.1 增量式Delaunay三角剖分算法
增量式Delaunay三角剖分算法是一种逐步构建Delaunay三角剖分的算法,其核心思想是逐个插入点,并对受影响的三角形进行调整,以保持Delaunay三角剖分的性质。
**算法步骤:**
1. 初始化一个空三角剖分。
2. 对于每个点p:
- 找到p最近的三角形T。
- 将p插入T,形成新的三角形集合T'。
- 对于T'中的每个三角形t:
- 如果t包含p的圆心,则删除t。
- 对于t的每个边e:
- 如果e与p的圆心相交,则将e分割成两条边。
3. 返回三角剖分。
**代码块:**
```python
def incremental_delaunay(points):
"""
增量式Delaunay三角剖分算法
参数:
points: 输入点集合
返回:
Delaunay三角剖分
"""
# 初始化空三角剖分
triangulation = []
# 逐个插入点
for p in points:
# 找到最近的三角形
t = find_nearest_triangle(triangulation, p)
# 插入点并形成新的三角形集合
t_prime = insert_point(t, p)
# 删除包含p的圆心的三角形
for t in t_prime:
if is_point_in_circle(t, p):
triangulation.remove(t)
# 分割与p的圆心相交的边
for t in t_prime:
for e in t.edges:
if is_edge_intersects_circle(e, p):
split_edge(e, p)
return triangulation
```
**逻辑分析:**
* `find_nearest_triangle` 函数找到距离给定点最近的三角形。
* `insert_point` 函数将点插入三角形,并形成新的三角形集合。
* `is_point_in_circle` 函数检查给定的点是否在三角形的圆心内。
* `split_edge` 函数将与给定点圆心相交的边分割成两条边。
### 3.2 Bowyer-Watson算法
Bowyer-Watson算法是另一种增量式Delaunay三角剖分算法,它使用Voronoi图来维护Delaunay三角剖分。
**算法步骤:**
1. 初始化一个空Voronoi图。
2. 对于每个点p:
- 创建p的Voronoi区域。
- 对于Voronoi区域的每个边e:
- 如果e与其他Voronoi区域的边f相交,则创建连接p和f的三角形。
3. 返回三角剖分。
**代码块:**
```python
def bowyer_watson(points):
"""
Bowyer-Watson算法
参数:
points: 输入点集合
返回:
Delaunay三角剖分
"""
# 初始化空Voronoi图
voronoi_diagram = []
# 逐个插入点
for p in points:
# 创建p的Voronoi区域
voronoi_region = create_voronoi_region(p)
# 对于Voronoi区域的每个边
for e in voronoi_region.edges:
# 如果e与其他Voronoi区域的边f相交
if is_edge_intersects_edge(e, f):
# 创建连接p和f的三角形
create_triangle(p, f)
return triangulation
```
**逻辑分析:**
* `create_voronoi_region` 函数创建给定点的Voronoi区域。
* `is_edge_intersects_edge` 函数检查两条边是否相交。
* `create_triangle` 函数创建连接两个点的三角形。
### 3.3 Delaunay三角剖分的优化算法
为了提高Delaunay三角剖分的效率,已经开发了各种优化算法。这些算法通常通过减少插入点或调整三角形集合来减少算法的时间复杂度。
**常见的优化算法:**
* **Delaunay翻转算法:**通过交换三角形的边来优化三角剖分。
* **最近邻搜索算法:**使用空间索引数据结构来快速找到最近的三角形。
* **并行算法:**利用多核处理器或分布式计算来并行化算法。
**表格:Delaunay三角剖分优化算法对比**
| 算法 | 时间复杂度 | 空间复杂度 |
|---|---|---|
| 增量式算法 | O(n^2) | O(n) |
| Bowyer-Watson算法 | O(n^2) | O(n) |
| Delaunay翻转算法 | O(n log n) | O(n) |
| 最近邻搜索算法 | O(n log n) | O(n) |
| 并行算法 | O(n) | O(n) |
# 4. Delaunay 三角剖分在 GIS 中的应用
Delaunay 三角剖分在 GIS 中具有广泛的应用,涉及地形建模、空间插值、路径规划和网络分析等领域。
### 4.1 地形建模和可视化
Delaunay 三角剖分可用于构建地形模型,通过连接一组不规则分布的点来创建连续的表面。该表面可用于可视化地形、计算坡度和坡向等地形属性。
**代码块:**
```python
import matplotlib.pyplot as plt
import scipy.spatial
# 创建点集
points = [(0, 0), (1, 1), (2, 2), (3, 3), (4, 4)]
# 构建 Delaunay 三角剖分
tri = scipy.spatial.Delaunay(points)
# 绘制 Delaunay 三角剖分
plt.triplot(points[:, 0], points[:, 1], tri.simplices)
plt.show()
```
**逻辑分析:**
* `scipy.spatial.Delaunay` 类用于构建 Delaunay 三角剖分。
* `tri.simplices` 属性包含三角剖分的索引,指示哪些点形成三角形。
* `plt.triplot` 函数绘制三角剖分。
### 4.2 空间插值和预测
Delaunay 三角剖分可用于执行空间插值,即根据已知点的数据预测未知点的数据。通过将未知点投影到最近的三角形,并使用三角形内点的加权平均值来估计未知点的值。
**代码块:**
```python
import numpy as np
import scipy.spatial
# 创建点集和数据值
points = [(0, 0, 1), (1, 1, 2), (2, 2, 3), (3, 3, 4), (4, 4, 5)]
# 构建 Delaunay 三角剖分
tri = scipy.spatial.Delaunay(points[:, :2])
# 预测未知点的值
unknown_point = (2.5, 2.5)
idx = tri.find_simplex(unknown_point)
weights = tri.transform[idx, :3]
value = np.dot(weights, points[tri.simplices[idx, :3], 2])
print("预测值:", value)
```
**逻辑分析:**
* `tri.find_simplex` 函数找到包含未知点的三角形。
* `tri.transform` 属性包含三角形变换矩阵,用于计算未知点的权重。
* `np.dot` 函数计算权重和已知点值的点积,得到预测值。
### 4.3 路径规划和网络分析
Delaunay 三角剖分可用于路径规划和网络分析,通过将空间网络表示为 Delaunay 三角剖分,并使用三角形边作为网络边。这允许使用最短路径算法(如 Dijkstra 算法)找到网络中的最优路径。
**代码块:**
```python
import networkx as nx
import scipy.spatial
# 创建点集和边
points = [(0, 0), (1, 1), (2, 2), (3, 3), (4, 4)]
edges = [(0, 1), (1, 2), (2, 3), (3, 4)]
# 构建 Delaunay 三角剖分
tri = scipy.spatial.Delaunay(points)
# 创建网络
G = nx.Graph()
for edge in edges:
G.add_edge(points[edge[0]], points[edge[1]])
# 构建 Delaunay 网络
delaunay_network = nx.Graph()
for triangle in tri.simplices:
for i in range(3):
delaunay_network.add_edge(points[triangle[i]], points[triangle[(i+1)%3]])
# 查找最短路径
path = nx.shortest_path(delaunay_network, points[0], points[4])
print("最短路径:", path)
```
**逻辑分析:**
* `networkx` 库用于创建和操作网络。
* `delaunay_network` 网络由 Delaunay 三角剖分的三角形边构成。
* `nx.shortest_path` 函数找到网络中两点之间的最短路径。
# 5. Delaunay 三角剖分的实践案例
### 5.1 使用 ArcGIS 构建 Delaunay 三角剖分
**步骤 1:加载数据**
在 ArcGIS 中,打开要进行 Delaunay 三角剖分的点要素图层。
**步骤 2:创建 Delaunay 三角剖分**
右键单击图层,选择“地理处理”>“分析工具”>“3D 分析”>“创建 Delaunay 三角剖分”。
**步骤 3:设置参数**
在“创建 Delaunay 三角剖分”工具对话框中,设置以下参数:
- 输入要素:要进行 Delaunay 三角剖分的点要素图层
- 输出 Delaunay 三角剖分:要创建的 Delaunay 三角剖分要素类
- 场:用于计算 Delaunay 三角剖分的 Z 值字段(可选)
**步骤 4:运行工具**
单击“确定”运行工具。工具将创建 Delaunay 三角剖分要素类,其中包含三角形要素,代表点之间的连接。
### 5.2 使用 Python 实现 Delaunay 三角剖分
**代码块 1:导入必要的库**
```python
import numpy as np
import scipy.spatial
```
**代码块 2:生成点集**
```python
# 生成 100 个随机点
points = np.random.rand(100, 2)
```
**代码块 3:创建 Delaunay 三角剖分**
```python
# 使用 SciPy 的 Delaunay 类创建 Delaunay 三角剖分
tri = scipy.spatial.Delaunay(points)
```
**代码块 4:获取三角形**
```python
# 获取三角形顶点的索引
triangles = tri.simplices
# 获取三角形顶点的坐标
triangle_points = points[triangles]
```
**代码块 5:绘制 Delaunay 三角剖分**
```python
import matplotlib.pyplot as plt
# 绘制点和三角形
plt.scatter(points[:, 0], points[:, 1])
for triangle in triangles:
plt.plot(triangle_points[triangle, :, 0], triangle_points[triangle, :, 1])
```
**逻辑分析:**
代码块 1 导入必要的库。代码块 2 生成一个包含 100 个随机点的点集。代码块 3 使用 SciPy 的 Delaunay 类创建 Delaunay 三角剖分。代码块 4 获取三角形的顶点索引和坐标。代码块 5 使用 Matplotlib 绘制点和三角形。
# 6. Delaunay三角剖分的发展趋势
### 6.1 大数据时代的Delaunay三角剖分
随着数据量的爆炸式增长,大数据时代的到来对Delaunay三角剖分提出了新的挑战。传统算法在处理海量数据时效率低下,因此需要探索新的算法和技术来应对大数据挑战。
一种方法是采用并行算法,通过将计算任务分配给多个处理单元,提高算法效率。另一种方法是使用近似算法,在保证一定精度的前提下,降低算法复杂度。
### 6.2 云计算和并行计算中的Delaunay三角剖分
云计算和并行计算的兴起为Delaunay三角剖分提供了新的机遇。云计算平台提供了弹性的计算资源,可以根据需要动态分配和释放资源。并行计算技术可以充分利用云计算平台的优势,实现大规模并行计算。
通过将Delaunay三角剖分算法移植到云计算平台,可以充分利用云计算的弹性资源和并行计算能力,显著提高算法效率。例如,可以使用Apache Spark等分布式计算框架,将Delaunay三角剖分算法并行化,从而实现大规模数据的快速处理。
此外,云计算平台还提供了丰富的存储和数据管理服务,可以方便地存储和管理海量数据,为Delaunay三角剖分算法的应用提供了便利。
0
0
相关推荐
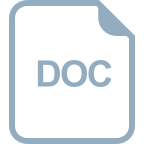
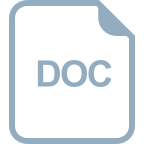
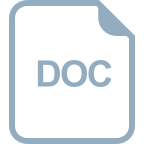
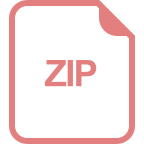
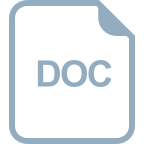
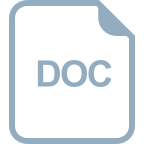
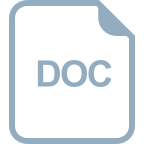
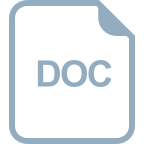
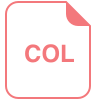