将决策树算法应用于含噪声的sin函数的回归问题,并可视化
时间: 2023-06-09 08:05:27 浏览: 105
答案:
决策树算法可以应用于含噪声的sin函数的回归问题。为了可视化决策树算法的应用与效果,可以使用Python中的Scikit-learn库进行实现。
首先,需要生成带有噪声的sin函数数据,可以使用如下代码:
```
import numpy as np
import matplotlib.pyplot as plt
# 生成含有噪声的sin函数数据
np.random.seed(0)
X = np.sort(5 * np.random.rand(80, 1), axis=0)
y = np.sin(X).ravel()
y[::5] += 3 * (0.5 - np.random.rand(16))
# 可视化生成的数据
plt.scatter(X, y, s=20, edgecolor="black",c="darkorange", alpha=0.5)
plt.show()
```
接着,需要使用Scikit-learn库中的DecisionTreeRegressor类进行决策树回归分析,可以使用如下代码:
```
from sklearn.tree import DecisionTreeRegressor
# 使用决策树回归分析
regr_1 = DecisionTreeRegressor(max_depth=2)
regr_2 = DecisionTreeRegressor(max_depth=5)
regr_3 = DecisionTreeRegressor(max_depth=8)
regr_1.fit(X, y)
regr_2.fit(X, y)
regr_3.fit(X, y)
# 生成测试数据
X_test = np.arange(0.0, 5.0, 0.01)[:, np.newaxis]
# 预测结果
y_1 = regr_1.predict(X_test)
y_2 = regr_2.predict(X_test)
y_3 = regr_3.predict(X_test)
```
最后,可以将回归结果可视化,使用如下代码:
```
plt.figure()
plt.scatter(X, y, s=20, edgecolor="black", c="darkorange", alpha=0.5, label="data")
plt.plot(X_test, y_1, color="cornflowerblue", label="max_depth=2", linewidth=2)
plt.plot(X_test, y_2, color="yellowgreen", label="max_depth=5", linewidth=2)
plt.plot(X_test, y_3, color="r", label="max_depth=8", linewidth=2)
plt.xlabel("data")
plt.ylabel("target")
plt.title("Decision Tree Regression")
plt.legend()
plt.show()
```
这样就可以得到含噪声的sin函数的回归问题的决策树回归分析可视化结果。
阅读全文
相关推荐




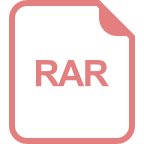









