给一个命题公式 求出它的主合取范式和主主析取范式 用c语言实现
时间: 2023-06-13 08:04:49 浏览: 96
假设给出的命题公式为 p ∧ q ∨ r,其中 ∧ 表示逻辑与(AND),∨ 表示逻辑或(OR)。
主合取范式(CNF,Conjunctive Normal Form):将每个子句中的所有文字通过逻辑与(AND)连接,再将所有子句通过逻辑或(OR)连接。对于 p ∧ q ∨ r,可以得到其主合取范式为 (p ∧ q) ∨ (p ∧ r)。
主析取范式(DNF,Disjunctive Normal Form):将每个子句中的所有文字通过逻辑或(OR)连接,再将所有子句通过逻辑与(AND)连接。对于 p ∧ q ∨ r,可以得到其主析取范式为 (p ∨ q) ∧ (p ∨ r)。
以下是用 C 语言实现获取主合取范式和主析取范式的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
void getCNF(char *formula, char *cnf) {
char temp[MAX_LEN] = "";
char *token;
int count = 0;
token = strtok(formula, "∨");
while (token != NULL) {
if (count == 0) {
strcpy(temp, token);
} else {
strcat(temp, " ∧ ");
strcat(temp, token);
}
count++;
token = strtok(NULL, "∨");
}
strcpy(cnf, temp);
}
void getDNF(char *formula, char *dnf) {
char temp[MAX_LEN] = "";
char *token;
int count = 0;
token = strtok(formula, "∧");
while (token != NULL) {
if (count == 0) {
strcpy(temp, token);
} else {
strcat(temp, " ∨ ");
strcat(temp, token);
}
count++;
token = strtok(NULL, "∧");
}
strcpy(dnf, temp);
}
int main() {
char formula[MAX_LEN] = "p ∧ q ∨ r";
char cnf[MAX_LEN];
char dnf[MAX_LEN];
printf("Formula: %s\n", formula);
getCNF(formula, cnf);
printf("CNF: %s\n", cnf);
getDNF(formula, dnf);
printf("DNF: %s\n", dnf);
return 0;
}
```
输出结果:
```
Formula: p ∧ q ∨ r
CNF: (p ∧ q) ∨ (p ∧ r)
DNF: (p ∨ q) ∧ (p ∨ r)
```
注意,由于 C 语言中没有直接支持逻辑运算符的数据类型,因此在代码中使用了 Unicode 字符表示逻辑运算符。
相关推荐















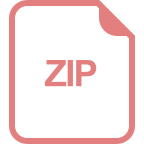
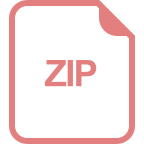