C语言数据结构基于顺序存储结构的图书信息表的创建和输出实现以下功能: 1.创建空顺序 2.输入图书信息 3.位置查找 4.价格查找 5.图书名查找 6.插入 7.删除 8.输出 0.退出
时间: 2024-10-21 17:08:32 浏览: 75
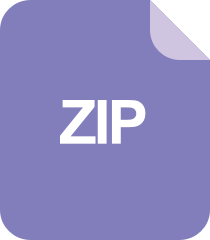
C语言期末大作业:用顺序存储结构实现图书管理系统

在C语言中,我们可以使用数组或动态内存分配(如`malloc()`函数)来创建基于顺序存储结构的图书信息表。这里是一个简单的示例,我们将使用结构体(struct)来表示图书,并通过数组实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义图书信息结构
typedef struct {
char title[100]; // 图书名称
float price; // 价格
} Book;
Book* createEmptyTable(int capacity) {
Book* table = (Book*)malloc(capacity * sizeof(Book));
if (!table) {
printf("Memory allocation failed.\n");
return NULL;
}
for (int i = 0; i < capacity; i++) {
table[i].title[0] = '\0'; // 初始化为结束符
table[i].price = 0.0f;
}
return table;
}
void inputBook(Book* table, int index) {
printf("Enter book information (title, price): ");
fgets(table[index].title, sizeof(table[index].title), stdin);
size_t len = strlen(table[index].title);
if (len > 0 && table[index].title[len - 1] == '\n') { // remove newline
table[index].title[len - 1] = '\0';
}
scanf("%f", &table[index].price);
}
void searchBook(Book* table, const char* title, float price) {
for (int i = 0; i < numBooks; i++) {
if (strcmp(table[i].title, title) == 0 && table[i].price == price) {
printf("Found book: %s, Price: %.2f\n", title, price);
break;
}
}
}
// 其他操作类似,例如 insert、delete 和 output 可以添加相应函数
int main() {
int capacity, numBooks = 0;
printf("Enter the capacity of the book table: ");
scanf("%d", &capacity);
Book* books = createEmptyTable(capacity);
if (!books) {
return 1;
}
while (1) {
printf("\n1. Add a book\n2. Search by title\n3. Search by price\n4. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
numBooks++;
if (numBooks >= capacity) {
printf("No more space left.\n");
continue;
}
inputBook(books, numBooks - 1);
break;
// ...其他选项的处理...
case 0:
free(books);
return 0;
}
// 输出书籍列表
printf("\nBooks in the table:\n");
for (int i = 0; i < numBooks; i++) {
printf("Title: %s, Price: %.2f\n", books[i].title, books[i].price);
}
}
}
```
在这个例子中,我们首先创建了一个可以存放一定数量图书的空表,然后允许用户选择不同的操作。注意,这个示例并没有处理所有可能的错误情况,实际应用中需要完善。
阅读全文
相关推荐
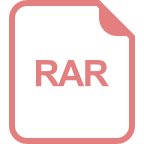
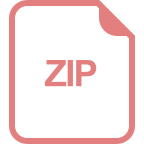






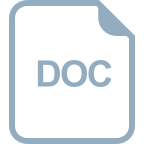








