实现图书信息管理,具体要求如下: 定义图书结构体类型ElemType,至少包括三个属性(书号,书名,价格);定义图书顺序表SqList(ElemType指针,表长,容量)。 功能:1、初始化创建一个空表(提示:需要分配存储空间,第二种顺序表类型定义);2、插入一条新的图书信息成为表中第i个元素;3、删除表中第i条图书信息;4、按书名进行查找,并返回且输出;5、查找表中的第i条图书信息;6、输出顺序表中的所有图书信息(注意排版清楚美观);7、修改指定图书信息的价格;8、自己分析,扩展有意义的功能。 主函数中定义顺序表变量L,通过调用各功能函数顺序完成以下操作:1.初始化创建空表L,2.连续插入3条数据到表头,3. 输出顺序表,4. 插入一条数据使其成为表中第3条数据,5. 输出顺序表,6. 根据指定的书名进行删除; 7. 输出顺序表; 8. 按书名进行查找,并输出查找到的图书完整信息; 9. 按书名查找后进行对应记录价格的修改; 10 .输出修改后全部图书信息。
时间: 2023-03-29 10:01:31 浏览: 62
ElemType结构体类型定义:
```
typedef struct {
int bookID; // 书号
char bookName[50]; // 书名
float price; // 价格
} ElemType;
```
图书顺序表SqList定义:
```
typedef struct {
ElemType *elem; // ElemType指针
int length; // 表长
int capacity; // 容量
} SqList;
```
1. 初始化创建一个空表
```
void InitList(SqList *L, int capacity) {
L->elem = (ElemType *)malloc(capacity * sizeof(ElemType));
L->length = ;
L->capacity = capacity;
}
```
2. 插入一条新的图书信息成为表中第i个元素
```
bool ListInsert(SqList *L, int i, ElemType e) {
if (i < 1 || i > L->length + 1 || L->length == L->capacity) {
return false;
}
for (int j = L->length; j >= i; j--) {
L->elem[j] = L->elem[j - 1];
}
L->elem[i - 1] = e;
L->length++;
return true;
}
```
3. 删除表中第i条图书信息
```
bool ListDelete(SqList *L, int i) {
if (i < 1 || i > L->length) {
return false;
}
for (int j = i; j < L->length; j++) {
L->elem[j - 1] = L->elem[j];
}
L->length--;
return true;
}
```
4. 按书名进行查找,并返回且输出
```
void FindBookByName(SqList *L, char *bookName) {
bool found = false;
for (int i = ; i < L->length; i++) {
if (strcmp(L->elem[i].bookName, bookName) == ) {
printf("书号:%d,书名:%s,价格:%.2f\n", L->elem[i].bookID, L->elem[i].bookName, L->elem[i].price);
found = true;
}
}
if (!found) {
printf("未找到书名为%s的图书\n", bookName);
}
}
```
5. 查找表中的第i条图书信息
```
bool GetElem(SqList *L, int i, ElemType *e) {
if (i < 1 || i > L->length) {
return false;
}
*e = L->elem[i - 1];
return true;
}
```
6. 输出顺序表中的所有图书信息
```
void PrintList(SqList *L) {
printf("图书信息如下:\n");
for (int i = ; i < L->length; i++) {
printf("书号:%d,书名:%s,价格:%.2f\n", L->elem[i].bookID, L->elem[i].bookName, L->elem[i].price);
}
}
```
7. 修改指定图书信息的价格
```
bool ModifyPrice(SqList *L, int bookID, float newPrice) {
bool found = false;
for (int i = ; i < L->length; i++) {
if (L->elem[i].bookID == bookID) {
L->elem[i].price = newPrice;
found = true;
}
}
return found;
}
```
8. 扩展功能:按价格排序并输出
```
void SortListByPrice(SqList *L) {
for (int i = ; i < L->length - 1; i++) {
for (int j = i + 1; j < L->length; j++) {
if (L->elem[i].price > L->elem[j].price) {
ElemType temp = L->elem[i];
L->elem[i] = L->elem[j];
L->elem[j] = temp;
}
}
}
PrintList(L);
}
```
主函数中的操作:
```
int main() {
SqList L;
InitList(&L, 10);
ElemType e1 = {1, "C语言程序设计", 29.9};
ElemType e2 = {2, "数据结构与算法", 49.9};
ElemType e3 = {3, "计算机组成原理", 39.9};
ListInsert(&L, 1, e1);
ListInsert(&L, 1, e2);
ListInsert(&L, 1, e3);
PrintList(&L);
ElemType e4 = {4, "操作系统", 59.9};
ListInsert(&L, 3, e4);
PrintList(&L);
ListDelete(&L, 2);
PrintList(&L);
FindBookByName(&L, "数据结构与算法");
ModifyPrice(&L, 1, 35.9);
PrintList(&L);
SortListByPrice(&L);
return ;
}
```
相关推荐
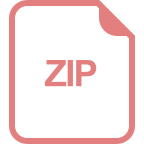
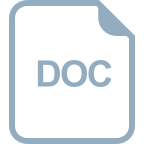
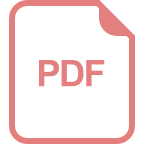














