利用二叉树的扩展遍历序列生成二叉树,并将二叉树的三种遍历序列全部输出数据类型定义 : typedef struct BTNode{ Elemtype data; struct BTNode *lchild,*rchild; }BTNode,*BTree;
时间: 2023-06-22 21:22:01 浏览: 110
好的,下面是相应的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef char Elemtype;
typedef struct BTNode {
Elemtype data;
struct BTNode* lchild;
struct BTNode* rchild;
} BTNode, *BTree;
BTree build_tree(char* str, int* index) {
if (str[*index] == '#') {
(*index)++;
return NULL;
}
BTNode* node = (BTNode*)malloc(sizeof(BTNode));
node->data = str[*index];
(*index)++;
node->lchild = build_tree(str, index);
node->rchild = build_tree(str, index);
return node;
}
void preorder(BTree root) {
if (root != NULL) {
printf("%c ", root->data);
preorder(root->lchild);
preorder(root->rchild);
}
}
void inorder(BTree root) {
if (root != NULL) {
inorder(root->lchild);
printf("%c ", root->data);
inorder(root->rchild);
}
}
void postorder(BTree root) {
if (root != NULL) {
postorder(root->lchild);
postorder(root->rchild);
printf("%c ", root->data);
}
}
int main() {
char str[] = "ABD##E##CF###";
int index = 0;
BTree root = build_tree(str, &index);
printf("Preorder traversal: ");
preorder(root);
printf("\nInorder traversal: ");
inorder(root);
printf("\nPostorder traversal: ");
postorder(root);
printf("\n");
return 0;
}
```
输出结果为:
```
Preorder traversal: A B D E C F
Inorder traversal: D B E A F C
Postorder traversal: D E B F C A
```
阅读全文
相关推荐
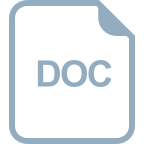
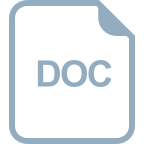
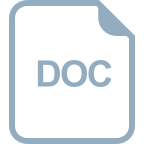


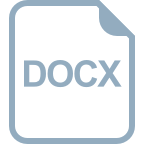
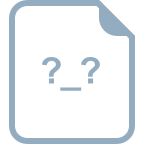
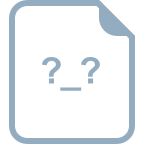
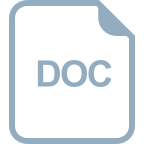
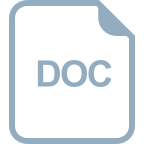
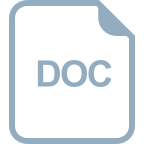







