已知结构体定义如下:typedef struct btonde{ElemTYpe element;struct btonde *lChild;struct btonde *rChild;}BTNode; 完成二叉树的先序创建、先序遍历、中序遍历、后序遍历等操作
时间: 2024-11-03 09:13:02 浏览: 35
在这个结构体 `BTNode` 定义中,`element` 表示元素类型,`lChild` 和 `rChild` 分别表示二叉树的左孩子和右孩子的指针。要完成二叉树的操作,我们可以按照如下的步骤:
### 1. **二叉树的先序创建 (Pre-order Construction)**
- 先插入根节点,然后递归地为左子树和右子树创建节点。
```c
void createPreOrder(BTNode **root, void* data) {
if (*root == NULL) {
*root = (BTNode*)malloc(sizeof(BTNode));
(*root)->element = data;
(*root)->lChild = NULL;
(*root)->rChild = NULL;
} else {
createPreOrder(&(*root)->lChild, data);
createPreOrder(&(*root)->rChild, data);
}
}
```
### 2. **先序遍历 (Pre-order Traversal)**
- 使用递归,先访问根节点,再遍历左子树和右子树。
```c
void preOrder(BTNode* node) {
if (node != NULL) {
printf("%p ", node->element); // 打印节点元素
preOrder(node->lChild);
preOrder(node->rChild);
}
}
```
### 3. **中序遍历 (In-order Traversal)**
- 先遍历左子树,访问根节点,再遍历右子树。
```c
void inOrder(BTNode* node) {
if (node != NULL) {
inOrder(node->lChild);
printf("%p ", node->element); // 打印节点元素
inOrder(node->rChild);
}
}
```
### 4. **后序遍历 (Post-order Traversal)**
- 先遍历左子树和右子树,最后访问根节点。
```c
void postOrder(BTNode* node) {
if (node != NULL) {
postOrder(node->lChild);
postOrder(node->rChild);
printf("%p ", node->element); // 打印节点元素
}
}
```
**相关问题--:**
1. 这些遍历方法的时间复杂度是多少?
2. 在实际应用中,如何选择先序、中序还是后序遍历?
3. 如果二叉树已经是倒置的,上述哪些遍历会受到影响?
阅读全文
相关推荐
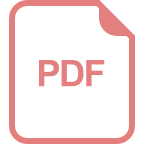
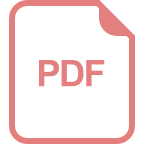
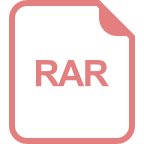





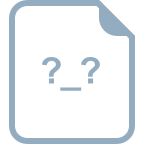
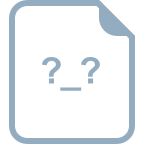
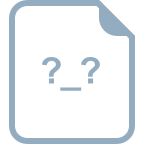
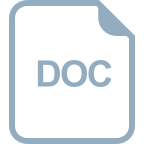
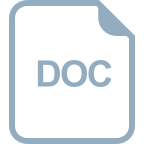





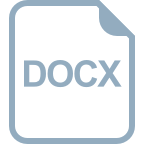