用c语言写一个把char字符转换成整型,然后存入数组的并用in读取的
时间: 2024-03-24 14:41:18 浏览: 117
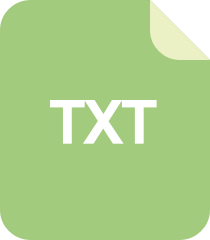
统计单词个数&&将字符串中连续出现的数字放进一维数组

好的,代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
const int MAX_LEN = 100;
char str[MAX_LEN];
int num[MAX_LEN];
int n;
printf("请输入字符串长度:");
scanf("%d", &n);
printf("请输入字符串:");
scanf("%s", str);
for (int i = 0; i < n; i++) {
num[i] = str[i] - '0'; // 将字符转换成整型
}
printf("转换成整型后的数组为:");
for (int i = 0; i < n; i++) {
printf("%d ", num[i]);
}
return 0;
}
```
该程序与之前的 C++ 版本类似,只是使用了 C 标准库中的 printf 和 scanf 函数。在读取字符串时,我们使用了 %s 占位符,表示读取一个字符串。另外,由于在 C 语言中没有 bool 类型,因此我们使用了 int 类型代替 bool 类型。
阅读全文
相关推荐
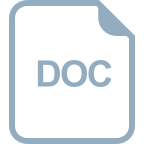
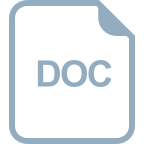


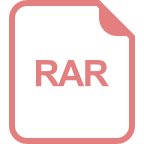
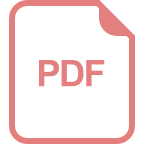
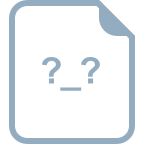










