微信小程序 切水果效果
时间: 2023-09-16 13:10:24 浏览: 77
微信小程序的切水果效果可以通过使用Canvas来实现。以下是一个简单的实现步骤:
1. 在小程序wxml文件中添加Canvas组件,设置Canvas的id和宽高:
```
<canvas id="fruit-canvas" style="width: 100%; height: 100%;"></canvas>
```
2. 在小程序js文件中获取Canvas对象,并设置Canvas的上下文:
```
const fruitCanvas = wx.createCanvasContext('fruit-canvas');
```
3. 创建一个Fruit对象来表示水果,包括水果的位置、大小、颜色、速度、方向等属性:
```
class Fruit {
constructor(x, y, size, color, speed, direction) {
this.x = x;
this.y = y;
this.size = size;
this.color = color;
this.speed = speed;
this.direction = direction;
}
draw() {
fruitCanvas.beginPath();
fruitCanvas.arc(this.x, this.y, this.size, 0, 2 * Math.PI);
fruitCanvas.fillStyle = this.color;
fruitCanvas.fill();
fruitCanvas.closePath();
}
move() {
if (this.direction === 'up') {
this.y -= this.speed;
} else if (this.direction === 'down') {
this.y += this.speed;
} else if (this.direction === 'left') {
this.x -= this.speed;
} else if (this.direction === 'right') {
this.x += this.speed;
}
}
}
```
4. 创建一个数组来保存所有的水果对象:
```
const fruits = [];
```
5. 在Canvas的touchstart、touchmove、touchend事件中,根据手指的移动距离和方向来切割水果:
```
let startX, startY;
function onTouchStart(event) {
startX = event.touches[0].clientX;
startY = event.touches[0].clientY;
}
function onTouchMove(event) {
const endX = event.touches[0].clientX;
const endY = event.touches[0].clientY;
const distanceX = endX - startX;
const distanceY = endY - startY;
fruits.forEach((fruit) => {
if (fruit.x - fruit.size < endX && fruit.x + fruit.size > startX &&
fruit.y - fruit.size < endY && fruit.y + fruit.size > startY) {
const angle = Math.atan2(distanceY, distanceX);
const speed = Math.sqrt(distanceX * distanceX + distanceY * distanceY) / 10;
const direction1 = {
x: Math.cos(angle - Math.PI / 4),
y: Math.sin(angle - Math.PI / 4),
};
const direction2 = {
x: Math.cos(angle + Math.PI / 4),
y: Math.sin(angle + Math.PI / 4),
};
const pieces = [];
pieces.push(new Fruit(fruit.x, fruit.y, fruit.size / 2, fruit.color, fruit.speed, direction1));
pieces.push(new Fruit(fruit.x, fruit.y, fruit.size / 2, fruit.color, fruit.speed, direction2));
fruits.splice(fruits.indexOf(fruit), 1);
fruits.push(...pieces);
}
});
startX = endX;
startY = endY;
}
function onTouchEnd(event) {
startX = null;
startY = null;
}
```
6. 在Canvas的定时器中,不断更新水果的位置和绘制水果:
```
setInterval(() => {
fruitCanvas.clearRect(0, 0, window.innerWidth, window.innerHeight);
fruits.forEach((fruit) => {
fruit.move();
fruit.draw();
});
}, 30);
```
这样就可以实现一个简单的切水果效果了。当然,还可以添加音效、分数等功能来丰富游戏体验。
相关推荐
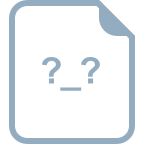
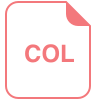
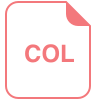
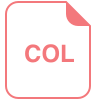
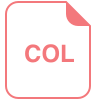
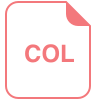









