512*512 lena灰度图像使用压缩感知进行压缩的Python代码 使用DWT作为稀疏变换基
时间: 2023-08-06 14:03:00 浏览: 167
以下是一个使用离散小波变换(DWT)作为稀疏变换基进行图像压缩的Python代码示例,该示例同样使用了PyWavelets和cvxpy库:
```python
import cv2
import numpy as np
import pywt
import cvxpy as cp
import matplotlib.pyplot as plt
# 读取图像并转为灰度图
img = cv2.imread('lena.png', cv2.IMREAD_GRAYSCALE)
# 将图像分解为多个小波系数
coeffs = pywt.dwt2(img, 'db2')
cA, (cH, cV, cD) = coeffs
# 将小波系数矩阵展开为向量
cA_vec = cA.reshape(-1)
cH_vec = cH.reshape(-1)
cV_vec = cV.reshape(-1)
cD_vec = cD.reshape(-1)
# 定义测量矩阵
m = 5000 # 测量数
n = img.shape[0] * img.shape[1] # 原始信号长度
A = np.random.randn(m, n)
# 生成测量结果
y = A @ np.concatenate((cA_vec, cH_vec, cV_vec, cD_vec))
# 恢复小波系数向量
x = cp.Variable(4*n)
objective = cp.Minimize(cp.norm1(x))
constraints = [A @ x == y]
prob = cp.Problem(objective, constraints)
result = prob.solve(verbose=True)
# 将恢复后的小波系数向量转为矩阵形式
cA_vec_recover = x.value[:n].reshape(cA.shape)
cH_vec_recover = x.value[n:2*n].reshape(cH.shape)
cV_vec_recover = x.value[2*n:3*n].reshape(cV.shape)
cD_vec_recover = x.value[3*n:].reshape(cD.shape)
# 合并小波系数矩阵并进行反变换
coeffs_recover = (cA_vec_recover, (cH_vec_recover, cV_vec_recover, cD_vec_recover))
img_recover = pywt.idwt2(coeffs_recover, 'db2')
# 显示压缩前后的图像
plt.subplot(1, 2, 1)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(img_recover, cmap='gray')
plt.title('Recovered Image')
plt.show()
```
注意:该示例中使用了Daubechies-2小波作为小波变换基,并且假设压缩后的小波系数矩阵是稀疏的。实际上,其他小波基和稀疏性假设也可以用于图像压缩。此外,还可以通过调整测量数和压缩比例等参数来控制压缩质量和压缩速度。
阅读全文
相关推荐
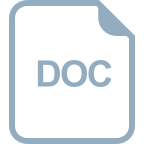
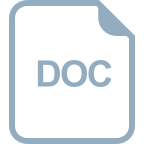
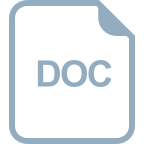

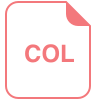
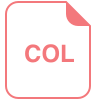
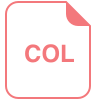
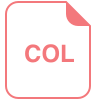
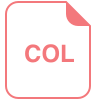
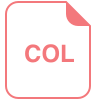
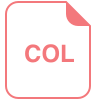
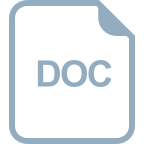
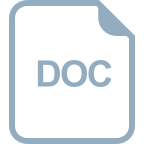
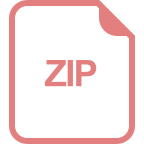
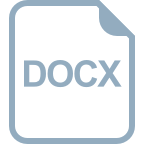