已知一个字符串s 设计一个算法来统计 s中字符出现的次数 用c语言编写
时间: 2024-05-11 07:15:13 浏览: 201
可以使用一个数组来存储每个字符出现的次数,遍历字符串s,对于s中的每个字符,将其对应的数组值加1。代码如下:
```c
#include <stdio.h>
#include <string.h>
void count_chars(char *s, int *count) {
int len = strlen(s);
for (int i = 0; i < len; i++) {
count[s[i]]++;
}
}
int main() {
char s[] = "Hello, world!";
int count[256] = {0};
count_chars(s, count);
for (int i = 0; i < 256; i++) {
if (count[i] > 0) {
printf("%c: %d\n", i, count[i]);
}
}
return 0;
}
```
输出结果为:
```
!: 1
,: 1
H: 1
e: 1
l: 3
o: 2
r: 1
w: 1
d: 1
```
注意,这个算法只能统计ASCII码中的字符,如果需要支持Unicode字符,需要使用更复杂的算法。
相关问题
C语言 已知一个字符串s,设计一个算法来统计字符串s中某个字符出现的次数
在C语言中,你可以使用标准库函数`strlen()`来计算字符串的长度,然后遍历整个字符串,用字符数组或者整型变量逐个计数目标字符。以下是一个简单的示例算法:
```c
#include <stdio.h>
#include <string.h>
int count_char(char *str, char target) {
int count = 0;
while (*str != '\0') { // 遍历直到字符串结束
if (*str == target) { // 如果当前字符等于目标字符
count++; // 增加计数
}
str++; // 移动到下一个字符
}
return count; // 返回字符出现的次数
}
int main() {
char s[] = "Hello, World!";
char target = 'o';
int result = count_char(s, target);
printf("字符 '%c' 出现了 %d 次.\n", target, result);
return 0;
}
```
在这个例子中,函数`count_char`接收一个字符串`str`和一个目标字符`target`,返回这个字符在字符串中出现的次数。
用C语言已知一个字符串s,设计一个算法来统计字符串s中某个字符出现的次数。
在C语言中,可以使用循环结构和数组或者直接利用`ctype.h`库中的`char`函数来统计字符串中某个字符出现的次数。这里提供两种简单的方法:
**方法一:遍历数组法**
```c
#include <stdio.h>
#include <string.h>
int count_char(char *s, char c) {
int count = 0;
while (*s != '\0') { // 遍历字符串直到遇到结束符
if (*s == c) {
count++;
}
s++; // 移动指针到下一个字符
}
return count;
}
int main() {
char str[] = "Hello, World!";
char target = 'o';
printf("字符 '%c' 在字符串 \"%s\" 中出现了 %d 次。\n", target, str, count_char(str, target));
return 0;
}
```
**方法二:利用`ctype.h`库**
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int count_char(char *s, char c) {
int count = 0;
while (*s) { // 遍历字符串直到遇到结束符
if (tolower(*s) == tolower(c)) { // 使用tolower处理大小写不敏感的情况
count++;
}
s++;
}
return count;
}
int main() {
char str[] = "Hello, World!";
char target = 'o';
printf("字符 '%c' (忽略大小写)在字符串 \"%s\" 中出现了 %d 次。\n", target, str, count_char(str, target));
return 0;
}
```
这两个例子都展示了如何统计给定字符串中特定字符的出现次数。
阅读全文
相关推荐
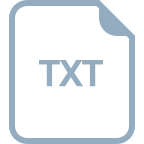
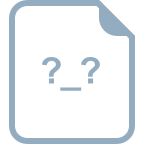
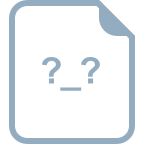









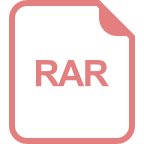



