已知一个字符串s,设计一个算法来统计字符串s中某个字符出现的次数。用c语言
时间: 2024-10-12 22:08:55 浏览: 49
在 C 语言中,你可以使用标准库函数 `strlen` 和一个循环来统计字符串中某个字符出现的次数。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
int count_char(const char* s, char target) {
int count = 0;
while (*s != '\0') { // 遍历整个字符串
if (*s == target) {
count++; // 如果当前字符等于目标字符,计数加一
}
s++; // 移动指针到下一个字符
}
return count; // 返回字符出现的次数
}
int main() {
char s[] = "Hello, World!";
char target = 'o';
int result = count_char(s, target);
printf("字符 '%c' 在字符串 '%s' 中出现了 %d 次.\n", target, s, result);
return 0;
}
```
这个程序首先检查字符串的每个字符,如果遇到目标字符,就增加计数器。当遍历完整个字符串后,返回计数器作为结果。
相关问题
C语言 已知一个字符串s,设计一个算法来统计字符串s中某个字符出现的次数
在C语言中,你可以使用标准库函数`strlen()`来计算字符串的长度,然后遍历整个字符串,用字符数组或者整型变量逐个计数目标字符。以下是一个简单的示例算法:
```c
#include <stdio.h>
#include <string.h>
int count_char(char *str, char target) {
int count = 0;
while (*str != '\0') { // 遍历直到字符串结束
if (*str == target) { // 如果当前字符等于目标字符
count++; // 增加计数
}
str++; // 移动到下一个字符
}
return count; // 返回字符出现的次数
}
int main() {
char s[] = "Hello, World!";
char target = 'o';
int result = count_char(s, target);
printf("字符 '%c' 出现了 %d 次.\n", target, result);
return 0;
}
```
在这个例子中,函数`count_char`接收一个字符串`str`和一个目标字符`target`,返回这个字符在字符串中出现的次数。
已知一个字符串s,设计一个算法统计串s中某个字符出现次数c语言
可以使用遍历字符串的方式,对每个字符进行比较,如果与目标字符相同,则计数器加1。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <string.h>
int countChar(char s[], char c) {
int count = 0;
for (int i = 0; i < strlen(s); i++) {
if (s[i] == c) {
count++;
}
}
return count;
}
int main() {
char s[1000];
char c;
printf("请输入字符串S:");
gets(s);
printf("请输入要统计的字符:");
scanf("%c", &c);
int count = countChar(s, c);
printf("字符%c在字符串S中出现了%d次。\n", c, count);
return 0;
}
```
阅读全文
相关推荐
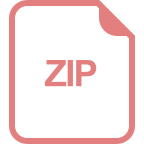
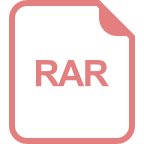
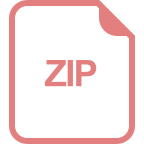













