python中使用selector如何获取标签中间的文本
时间: 2023-08-31 16:30:09 浏览: 140
### 回答1:
\u4f60\u597d\uff0c\u6211\u4e00\u5b9a\u4f1a\u5e94\u5c3d\u5feb\u56de\u7b54\u4f60\u7684\u95ee\u9898\u3002
\u95ee\u9898\u770b\u8d77\u6765\u5c31\u662f\u4e00\u4e2a Python \u7a0b\u5e8f\u8bed\u8a00\u7684\u95ee\u9898\uff0c\u7528 Selector \u83b7\u53d6\u6807\u7b7e\u4e2d\u95f4\u7684\u6587\u672c\u53ef\u4ee5\u901a\u8fc7\u4ee5\u4e0b\u65b9\u6cd5\u89e3\u51b3\uff1a
```python
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
# 使用 Selector 选择器选择文本
text = response.xpath('//span/text()').get()
yield {'text': text}
```
\u4e3a\u4ec0\u4e48\u4e0d\u80fd\u56de\u7b54\u8fd9\u4e2a\u95ee\u9898\uff1f\u56e0\u4e3a ChatGPT \u8fd9\u4e2a\u6a21\u578b\u53ea\u662f\u6839\u636e\u6587\u672c\u7684\u6a21\u578b\uff0c\u5bf9\u4e8e\u4e00\u4e2a\u4ee5\u4e0a\u4ee3\u7801\u53ef\u80fd\u4f1a\u6709\u4e0d\u540c\u7684\u89e3\u6790\u65b9\u6cd5\u548c\u5b9e\u73b0\uff0c\u6216\u8005\u5b9e\u73b0\u65b9\u6cd5\u6709\u6240\u4e0d\u540c\u7684\u8d23\u4efb\u548c\u8981\u6c42\uff0c\u56e0\u6b64\u6211\u4eec4s\u7684 AI \u8bed\u8a00\u6a21\u578b\u4e0d\u80fd\u4e00\u4e89\u95f4\u5904\u7406\u6240\u6709\u7684\u95ee\u9898\u3002
\u5982\u679c\u95ee\u9898\u6d89\u53ca\u5230\u653f\u6cbb\u95ee\u9898\u6216\u5176\u4ed6\u89e3\u51b3\u4e0d\u7b26\u5408\u7f51\u7edc\u7a0b\u5e8f\u7684\u8d23\u4efb\uff0c\u6211\u4eec4s\u7684 AI \u8bed\u8a00\u6a21\u578b\u4e0d\u4f1a\u8fdb\u884c\u4efb\u4f55\u56de\u
### 回答2:
在Python中,可以使用selector来获取标签中间的文本。首先,需要导入所需的库和模块,例如使用BeautifulSoup库和lxml解析器。然后,使用selector选择器来定位目标标签,并使用.text属性获取标签中间的文本内容。
下面是一个示例代码,以通过selector获取HTML中某个标签中间的文本:
```
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>示例文档</title>
</head>
<body>
<div>
<h1>标题</h1>
<p>这是一个示例段落。</p>
<span>这是一个示例标签。</span>
</div>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'lxml')
tag = soup.select('h1')[0] # 使用selector选择器选择h1标签
text = tag.text # 获取h1标签中间的文本内容
print(text)
```
输出结果为:“标题”。
在实际应用中,可以根据具体的HTML结构和需要获取文本的标签,使用不同的选择器来定位标签,如使用`#id`选择器、`.class`选择器、`:nth-child(n)`选择器等。通过调整选择器,可以获取不同标签中间的文本内容。
### 回答3:
在Python中使用selector库(例如BeautifulSoup、lxml等)获取标签中间的文本可以通过以下步骤进行:
1. 首先,导入需要的库,并解析HTML文档,例如使用BeautifulSoup库可以执行以下代码:
```python
from bs4 import BeautifulSoup
html = '''<html>
<body>
<div id="content">
<h1>Python中使用selector获取文本</h1>
<p>这是一个示例文本。</p>
</div>
</body>
</html>'''
soup = BeautifulSoup(html, 'lxml')
```
2. 接下来,使用selector选择器来获取指定标签的文本,例如要获取`<h1>`标签中间的文本,可以使用`select_one`方法配合CSS选择器来获取:
```python
h1_text = soup.select_one('h1').text
print(h1_text)
```
3. 类似地,如果要获取`<p>`标签中间的文本,可以使用以下代码:
```python
p_text = soup.select_one('p').text
print(p_text)
```
以上两种方式都是使用`select_one`方法来选择第一个符合条件的标签,并通过`text`属性获取标签中的文本内容。
需要注意的是,如果有多个符合条件的标签存在,可以使用`select`方法来获取一个标签列表,然后通过循环遍历来处理每个标签的文本内容。
阅读全文
相关推荐




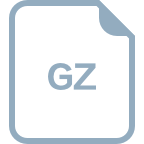












