Pytorch框架中的基础api的使用,
时间: 2024-02-09 10:08:54 浏览: 121
PyTorch是一个基于Python的科学计算库,它支持动态计算图,可用于创建深度学习模型。下面是PyTorch中一些基础API的使用:
1. 张量操作
张量是PyTorch中的基本数据结构,类似于NumPy中的数组。PyTorch中的张量操作与NumPy中的数组操作类似,可以进行加、减、乘、除等运算。
```python
import torch
# 创建张量
x = torch.tensor([[1, 2], [3, 4]])
y = torch.tensor([[5, 6], [7, 8]])
# 张量加法
z = x + y
# 张量乘法
z = torch.matmul(x, y)
# 张量转置
z = x.t()
# 张量求和
z = torch.sum(x)
```
2. 自动求导
PyTorch中的自动求导功能可以自动计算变量的梯度。为了使用自动求导功能,需要将变量设置为`requires_grad=True`。
```python
import torch
# 创建张量,并设置requires_grad=True
x = torch.tensor([1.0, 2.0], requires_grad=True)
y = torch.tensor([3.0, 4.0], requires_grad=True)
# 计算函数
z = x + y
w = z.sum()
# 计算梯度
w.backward()
# 变量梯度
print(x.grad)
print(y.grad)
```
3. 模型构建
PyTorch中可以使用`torch.nn`模块来构建神经网络模型。`torch.nn`中包含了多种层类型,如全连接层、卷积层、池化层等。
```python
import torch.nn as nn
# 定义模型类
class Model(nn.Module):
def __init__(self):
super(Model, self).__init__()
self.fc = nn.Linear(10, 5)
def forward(self, x):
x = self.fc(x)
return x
# 创建模型实例
model = Model()
# 计算输出
input = torch.randn(1, 10)
output = model(input)
```
以上是PyTorch中一些基础API的使用,希望对你有所帮助。
阅读全文
相关推荐
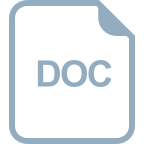
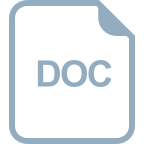
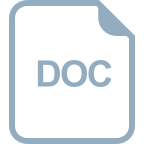
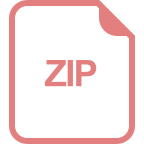
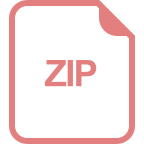
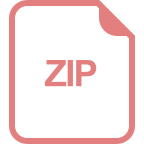
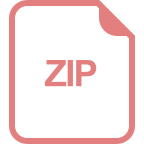
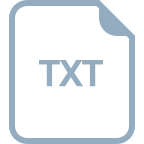
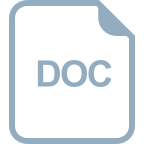
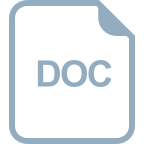
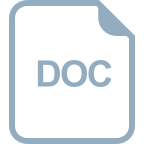
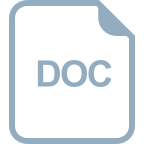






