编写程序以创建多项选择测验 需要一个基于 GUI 的程序,使人们能够从多项选择题库中进行测试。每个问题包括: 问题文本; 4个可能的答案; 正确答案的数量(1-4,而不是0-3); 问题按顺序保存在文本文件中,每个问题保存在 6 行上。问题文本文件中的示例条目可能如下所示: 该计划应具有以下特点: 一个包含“打开”和“退出”的菜单,其中“打开”启动 JFileChooser 以选择包含问题的文件,“退出”结束程序。 加载文件后,GUI 应一次显示一个问题及其答案。 用户应该能够选择一个答案,并且应该通知他们是否正确。 应让用户知道正确回答的数量和回答的问题总数。 用户应该只有在回答了当前问题后才能继续下一个问题。 回答完所有问题后,应通知用户他们的总分以及游戏已完成。现在应启用“打开”菜单项以启动新测验。(可选)您可以添加重新启动菜单项以重做当前测验。
时间: 2024-02-05 21:10:37 浏览: 81
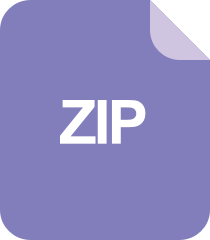
选择题程序

这是一个比较复杂的程序,需要用到 Java 的 GUI 编程。以下是一个简单的 Java 代码示例,可以实现上述功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
public class MultipleChoiceQuiz extends JFrame implements ActionListener {
private JMenuItem openMenuItem, restartMenuItem, exitMenuItem;
private JFileChooser fileChooser;
private JLabel questionLabel;
private JRadioButton answer1RadioButton, answer2RadioButton, answer3RadioButton, answer4RadioButton;
private ButtonGroup answerButtonGroup;
private JButton submitButton, nextButton;
private ArrayList<Question> questions;
private int currentQuestionIndex, correctAnswers, totalQuestions;
public MultipleChoiceQuiz() {
super("Multiple Choice Quiz");
// Create menu bar
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
openMenuItem = new JMenuItem("Open...");
openMenuItem.addActionListener(this);
restartMenuItem = new JMenuItem("Restart");
restartMenuItem.addActionListener(this);
exitMenuItem = new JMenuItem("Exit");
exitMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
fileMenu.add(restartMenuItem);
fileMenu.add(exitMenuItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// Create GUI components
questionLabel = new JLabel();
answer1RadioButton = new JRadioButton();
answer2RadioButton = new JRadioButton();
answer3RadioButton = new JRadioButton();
answer4RadioButton = new JRadioButton();
answerButtonGroup = new ButtonGroup();
answerButtonGroup.add(answer1RadioButton);
answerButtonGroup.add(answer2RadioButton);
answerButtonGroup.add(answer3RadioButton);
answerButtonGroup.add(answer4RadioButton);
submitButton = new JButton("Submit");
submitButton.addActionListener(this);
nextButton = new JButton("Next");
nextButton.addActionListener(this);
// Add components to content pane
Container contentPane = getContentPane();
contentPane.setLayout(new GridLayout(6, 1));
contentPane.add(questionLabel);
contentPane.add(answer1RadioButton);
contentPane.add(answer2RadioButton);
contentPane.add(answer3RadioButton);
contentPane.add(answer4RadioButton);
contentPane.add(submitButton);
pack();
// Create file chooser
fileChooser = new JFileChooser();
// Initialize variables
questions = new ArrayList<Question>();
currentQuestionIndex = 0;
correctAnswers = 0;
totalQuestions = 0;
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == openMenuItem) {
int returnVal = fileChooser.showOpenDialog(this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
String filename = fileChooser.getSelectedFile().getAbsolutePath();
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(";");
if (parts.length == 5) {
String questionText = parts[0];
String answer1Text = parts[1];
String answer2Text = parts[2];
String answer3Text = parts[3];
String answer4Text = parts[4];
Question question = new Question(questionText, answer1Text, answer2Text, answer3Text, answer4Text);
questions.add(question);
}
}
reader.close();
totalQuestions = questions.size();
displayQuestion();
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error reading file: " + ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
} else if (e.getSource() == restartMenuItem) {
currentQuestionIndex = 0;
correctAnswers = 0;
displayQuestion();
} else if (e.getSource() == exitMenuItem) {
System.exit(0);
} else if (e.getSource() == submitButton) {
if (answer1RadioButton.isSelected() && questions.get(currentQuestionIndex).isCorrectAnswer(1)) {
correctAnswers++;
} else if (answer2RadioButton.isSelected() && questions.get(currentQuestionIndex).isCorrectAnswer(2)) {
correctAnswers++;
} else if (answer3RadioButton.isSelected() && questions.get(currentQuestionIndex).isCorrectAnswer(3)) {
correctAnswers++;
} else if (answer4RadioButton.isSelected() && questions.get(currentQuestionIndex).isCorrectAnswer(4)) {
correctAnswers++;
}
displayResults();
nextButton.setEnabled(true);
submitButton.setEnabled(false);
} else if (e.getSource() == nextButton) {
currentQuestionIndex++;
if (currentQuestionIndex < totalQuestions) {
displayQuestion();
nextButton.setEnabled(false);
submitButton.setEnabled(true);
} else {
displayResults();
nextButton.setEnabled(false);
submitButton.setEnabled(false);
}
}
}
private void displayQuestion() {
Question question = questions.get(currentQuestionIndex);
questionLabel.setText(question.getQuestionText());
answer1RadioButton.setText(question.getAnswer1Text());
answer2RadioButton.setText(question.getAnswer2Text());
answer3RadioButton.setText(question.getAnswer3Text());
answer4RadioButton.setText(question.getAnswer4Text());
answerButtonGroup.clearSelection();
}
private void displayResults() {
int incorrectAnswers = totalQuestions - correctAnswers;
double score = ((double) correctAnswers / totalQuestions) * 100;
String message = String.format("You answered %d out of %d questions correctly (%.1f%%).",
correctAnswers, totalQuestions, score);
JOptionPane.showMessageDialog(this, message, "Results", JOptionPane.INFORMATION_MESSAGE);
}
private static class Question {
private String questionText;
private String answer1Text;
private String answer2Text;
private String answer3Text;
private String answer4Text;
private int numCorrectAnswers;
public Question(String questionText, String answer1Text, String answer2Text, String answer3Text, String answer4Text) {
this.questionText = questionText;
this.answer1Text = answer1Text;
this.answer2Text = answer2Text;
this.answer3Text = answer3Text;
this.answer4Text = answer4Text;
this.numCorrectAnswers = 1;
}
public String getQuestionText() {
return questionText;
}
public String getAnswer1Text() {
return answer1Text;
}
public String getAnswer2Text() {
return answer2Text;
}
public String getAnswer3Text() {
return answer3Text;
}
public String getAnswer4Text() {
return answer4Text;
}
public boolean isCorrectAnswer(int answerNumber) {
if (answerNumber == 1) {
return numCorrectAnswers == 1;
} else if (answerNumber == 2) {
return numCorrectAnswers == 2;
} else if (answerNumber == 3) {
return numCorrectAnswers == 3;
} else if (answerNumber == 4) {
return numCorrectAnswers == 4;
} else {
return false;
}
}
}
public static void main(String[] args) {
MultipleChoiceQuiz quiz = new MultipleChoiceQuiz();
quiz.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
quiz.setVisible(true);
}
}
```
这个程序的主要思路是在 `actionPerformed` 方法中处理各种事件(如菜单项点击、提交按钮点击、下一题按钮点击等)。当用户打开题目文件时,程序会读取文件并将每个问题存储在 `questions` 列表中。程序会跟踪当前题目索引 `currentQuestionIndex`,以及用户答对的题目数量 `correctAnswers` 和题目总数 `totalQuestions`。每次显示一个问题时,程序会将问题文本和答案选项显示在相应的 GUI 组件中,并清除之前的答案选择。当用户提交答案时,程序会检查选择的答案是否正确,并更新 `correctAnswers` 的值。在用户回答完所有的问题后,程序会弹出一个消息框显示用户的得分。
阅读全文
相关推荐
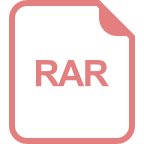
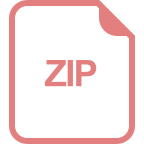

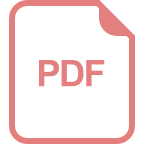
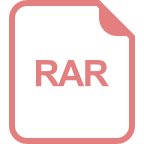
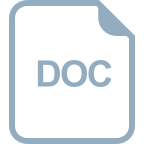
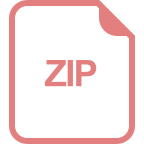
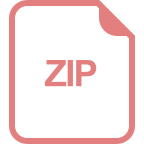
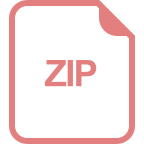
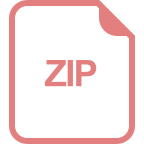
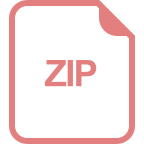
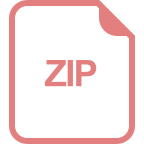
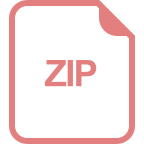
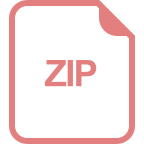
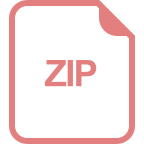