c语言使用fopen_s怎么求wav的时域信号功率谱
时间: 2024-05-12 08:16:32 浏览: 151
你可以使用以下代码来求wav的时域信号功率谱:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define BUFFER_SIZE 4096
int main(int argc, char* argv[])
{
if(argc < 2)
{
printf("Usage: %s <input_wav_file>\n", argv[0]);
return 1;
}
FILE* input_file;
errno_t err = fopen_s(&input_file, argv[1], "rb");
if(err != 0)
{
printf("Error opening input file: %s\n", argv[1]);
return 1;
}
char buffer[BUFFER_SIZE];
int bytes_read;
int num_samples = 0;
int sample_rate = 0;
short sample;
// Read the header of the WAV file
bytes_read = fread(buffer, sizeof(char), 44, input_file);
if(bytes_read != 44)
{
printf("Error reading WAV header\n");
fclose(input_file);
return 1;
}
// Parse the header to get the number of samples and the sample rate
num_samples = *(int*)(buffer + 40);
sample_rate = *(int*)(buffer + 24);
// Allocate memory for the audio data
short* audio_data = (short*)malloc(num_samples * sizeof(short));
if(audio_data == NULL)
{
printf("Error allocating memory for audio data\n");
fclose(input_file);
return 1;
}
// Read the audio data from the file
bytes_read = fread(audio_data, sizeof(short), num_samples, input_file);
if(bytes_read != num_samples)
{
printf("Error reading audio data\n");
free(audio_data);
fclose(input_file);
return 1;
}
fclose(input_file);
// Compute the power spectrum of the audio data
int num_bins = BUFFER_SIZE / 2;
double* power_spectrum = (double*)malloc(num_bins * sizeof(double));
if(power_spectrum == NULL)
{
printf("Error allocating memory for power spectrum\n");
free(audio_data);
return 1;
}
int window_size = BUFFER_SIZE;
int hop_size = BUFFER_SIZE / 2;
int num_frames = (num_samples - window_size) / hop_size + 1;
for(int i = 0; i < num_frames; i++)
{
int start_index = i * hop_size;
int end_index = start_index + window_size;
if(end_index > num_samples)
{
end_index = num_samples;
}
// Apply a window function to the audio data
for(int j = start_index; j < end_index; j++)
{
audio_data[j] *= 0.5 * (1 - cos(2 * M_PI * (j - start_index) / (window_size - 1)));
}
// Compute the FFT of the windowed audio data
double* real_part = (double*)malloc(window_size * sizeof(double));
double* imag_part = (double*)malloc(window_size * sizeof(double));
for(int j = 0; j < window_size; j++)
{
real_part[j] = audio_data[start_index + j];
imag_part[j] = 0;
}
fft(real_part, imag_part, window_size);
// Compute the power spectrum of the FFT
for(int j = 0; j < num_bins; j++)
{
double magnitude = sqrt(real_part[j] * real_part[j] + imag_part[j] * imag_part[j]);
power_spectrum[j] += magnitude * magnitude / (window_size * hop_size);
}
free(real_part);
free(imag_part);
}
// Free memory
free(audio_data);
// Print the power spectrum to stdout
for(int i = 0; i < num_bins; i++)
{
double frequency = (double)i * sample_rate / BUFFER_SIZE;
printf("%f %f\n", frequency, power_spectrum[i]);
}
free(power_spectrum);
return 0;
}
// Computes the FFT of the given real and imaginary arrays of length n
void fft(double* real, double* imag, int n)
{
if(n == 1)
{
return;
}
double* even_real = (double*)malloc(n / 2 * sizeof(double));
double* even_imag = (double*)malloc(n / 2 * sizeof(double));
double* odd_real = (double*)malloc(n / 2 * sizeof(double));
double* odd_imag = (double*)malloc(n / 2 * sizeof(double));
for(int i = 0; i < n / 2; i++)
{
even_real[i] = real[2 * i];
even_imag[i] = imag[2 * i];
odd_real[i] = real[2 * i + 1];
odd_imag[i] = imag[2 * i + 1];
}
fft(even_real, even_imag, n / 2);
fft(odd_real, odd_imag, n / 2);
for(int i = 0; i < n / 2; i++)
{
double angle = -2 * M_PI * i / n;
double cos_angle = cos(angle);
double sin_angle = sin(angle);
real[i] = even_real[i] + cos_angle * odd_real[i] - sin_angle * odd_imag[i];
imag[i] = even_imag[i] + cos_angle * odd_imag[i] + sin_angle * odd_real[i];
real[i + n / 2] = even_real[i] - cos_angle * odd_real[i] + sin_angle * odd_imag[i];
imag[i + n / 2] = even_imag[i] - cos_angle * odd_imag[i] - sin_angle * odd_real[i];
}
free(even_real);
free(even_imag);
free(odd_real);
free(odd_imag);
}
```
这个代码使用了一个缓冲区来读取WAV文件的数据,并对缓冲区中的数据进行窗口函数和FFT处理,然后计算功率谱。该代码还使用了一个自定义的FFT函数,用于计算实数和虚数数组的FFT。
阅读全文
相关推荐
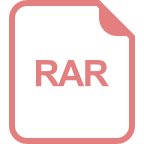

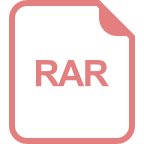
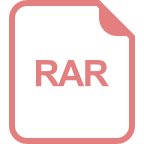
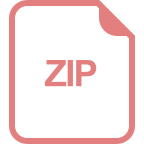
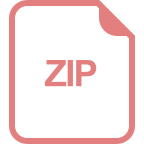
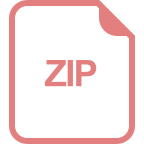
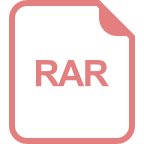
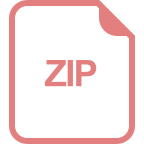
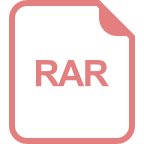
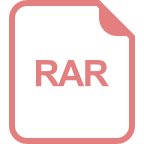
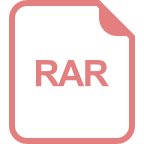
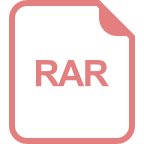
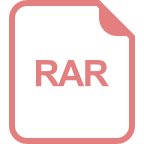
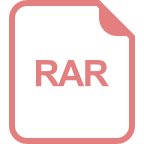
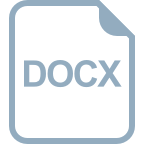
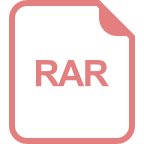