最短路问题非线性python编程代码
时间: 2023-09-22 09:12:28 浏览: 46
最短路问题是一个经典的图论问题,常见的算法有Dijkstra算法和Bellman-Ford算法。下面是一个非线性python编程代码,实现了Dijkstra算法来解决最短路问题:
```python
import heapq
def dijkstra(graph, start, end):
# 初始化距离字典和前驱节点字典
dist = {node: float('inf') for node in graph}
dist[start] = 0
prev = {node: None for node in graph}
# 采用堆优化的Dijkstra算法
pq = [(0, start)]
while pq:
current_dist, current_node = heapq.heappop(pq)
if current_dist > dist[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_dist + weight
if distance < dist[neighbor]:
dist[neighbor] = distance
prev[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
# 构造最短路径
path = []
node = end
while node is not None:
path.append(node)
node = prev[node]
path.reverse()
return path, dist[end]
```
其中,graph是一个字典,表示图的邻接矩阵,start和end是起点和终点。该代码使用堆优化的Dijkstra算法,具有较好的时间复杂度。
相关推荐
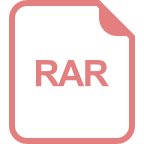













