C++异常以及错误处理使用try块简单代码演示
时间: 2024-10-22 09:25:08 浏览: 17
在C++中,异常处理是一种强大的机制,用于程序在运行期间遇到错误条件时恢复流程。当程序遇到预期之外的状况(例如除数为零、文件无法打开等),可以使用`try-catch`块来捕获并处理这些异常。下面是一个简单的代码示例:
```cpp
#include <iostream>
#include <exception>
// 自定义异常类型
class CustomException : public std::runtime_error {
public:
CustomException(const char* msg) : std::runtime_error(msg) {}
};
int main() {
try {
// 尝试执行可能会抛出异常的操作
int x = 5;
if (x == 0) {
throw CustomException("除数不能为零");
}
std::cout << "Dividing by " << x << "\n";
} catch (CustomException& e) {
// 捕获并处理自定义异常
std::cerr << "Caught a custom exception: " << e.what() << '\n';
} catch (std::exception& e) {
// 捕获所有标准异常
std::cerr << "Caught a standard exception: " << e.what() << '\n';
} catch (...) {
// 捕获所有未预见的异常
std::cerr << "Caught an unknown exception.\n";
}
return 0;
}
```
在这个例子中,如果变量`x`等于0,`throw`会抛出我们自定义的`CustomException`。然后,代码会在`catch`块中寻找匹配的异常类型,并执行相应的错误处理代码。如果没有特定的匹配,它将按顺序尝试更一般类型的异常。
阅读全文
相关推荐
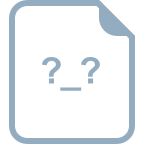
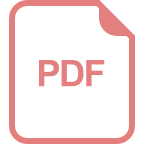
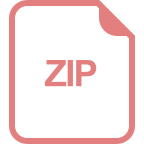

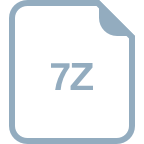
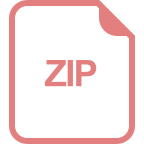
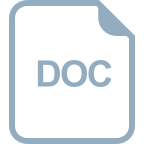
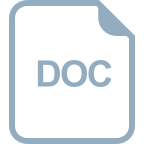
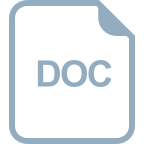
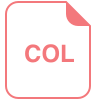
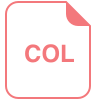
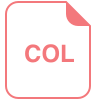
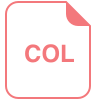
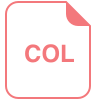


