c++异常处理使用try-catch块代码演示
时间: 2024-10-22 18:04:14 浏览: 29
在C++中,异常处理是一种错误处理机制,它通过`try-catch`块来捕获并处理程序运行期间可能出现的错误。下面是一个简单的`try-catch`块的代码示例:
```cpp
#include <iostream>
#include <exception>
// 定义一个自定义异常类
class CustomException : public std::runtime_error {
public:
CustomException(const char* message) : std::runtime_error(message) {}
};
int main() {
try {
// 尝试可能会抛出异常的代码
int result = divideByZero(10, 0);
std::cout << "Result: " << result << std::endl;
} catch (CustomException& e) { // 捕获我们自定义的异常
std::cerr << "Caught CustomException: " << e.what() << std::endl;
} catch (std::exception& e) { // 捕获所有标准异常
std::cerr << "Caught standard exception: " << e.what() << std::endl;
} catch (...) { // 捕获未知类型的所有异常
std::cerr << "Caught unknown exception" << std::endl;
}
return 0;
}
// 这里是一个可能会抛出异常的函数
int divideByZero(int numerator, int denominator) throw(CustomException) {
if (denominator == 0) {
throw CustomException("Division by zero is not allowed.");
}
return numerator / denominator;
}
```
在这个例子中,如果`divideByZero`函数的`denominator`为零,会抛出`CustomException`。`try`块包围了可能会引发异常的代码,如果发生异常,控制权就会转到相应的`catch`块中,根据异常类型进行适当的处理。
阅读全文
相关推荐













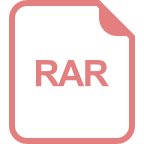




