用go语言实现比较月日时分,来判断是否大于当前系统时间
时间: 2024-09-23 21:07:10 浏览: 27
在Go语言中,你可以创建两个结构体,分别表示日期和时间,然后通过`time.Now()`获取当前系统的日期和时间。以下是一个简单的示例,展示如何实现这个功能:
```go
package main
import (
"fmt"
"time"
)
type DateTime struct {
Month int
Day int
Hour int
Minute int
}
func isFuture(dt DateTime) bool {
now := time.Now()
return now.Month() < dt.Month || (now.Month() == dt.Month && now.Day() < dt.Day) ||
(now.Month() == dt.Month && now.Day() == dt.Day && now.Hour() < dt.Hour) ||
(now.Month() == dt.Month && now.Day() == dt.Day && now.Hour() == dt.Hour && now.Minute() < dt.Minute)
}
func main() {
// 例如,我们想要检查的未来日期和时间
futureDateTime := DateTime{Month: 12, Day: 31, Hour: 23, Minute: 59}
if isFuture(futureDateTime) {
fmt.Printf("%d/%02d/%02d %02d:%02d 比当前时间更晚\n", futureDateTime.Month, futureDateTime.Day, futureDateTime.Hour, futureDateTime.Minute)
} else {
fmt.Printf("%d/%02d/%02d %02d:%02d 已经过去了或等于当前时间\n", futureDateTime.Month, futureDateTime.Day, futureDateTime.Hour, futureDateTime.Minute)
}
}
```
在这个例子中,`isFuture`函数会比较给定的日期时间是否大于当前时间。如果大于,则返回`true`,否则返回`false`。
阅读全文
相关推荐
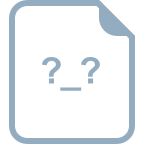
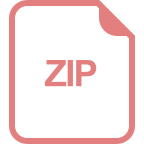
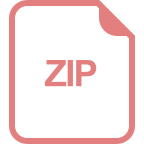


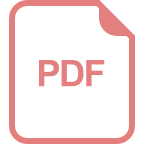
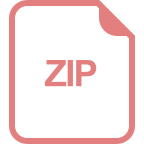
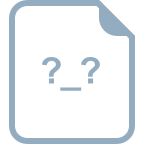
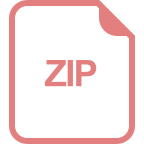
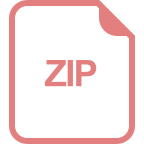
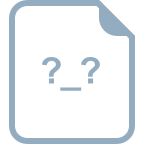
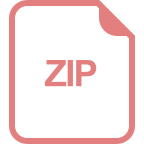
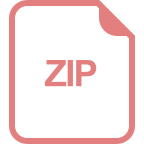
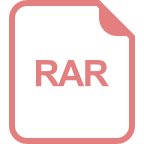
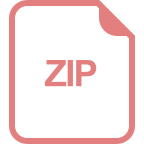
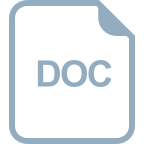
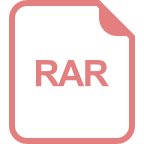
