qt调用v4l2
时间: 2023-07-05 09:11:39 浏览: 183

qt_v4l2.tar.gz_V4L2 qt_qt 采集_qt+v4l2_qt视频显示‘_v4l2 QT
可以使用 Qt 的多媒体框架(Qt Multimedia)来调用 V4L2 捕获摄像头的图像数据。以下是一个基本的 Qt 代码示例,可用于使用 V4L2 调用摄像头并显示实时图像:
```c++
#include <QtGui>
#include <QtMultimedia>
class Camera : public QObject
{
Q_OBJECT
public:
Camera(QObject *parent = nullptr);
virtual ~Camera();
void start();
void stop();
signals:
void imageCaptured(const QImage &image);
private slots:
void handleStateChanged(QCamera::State state);
void handleCapture(const QVideoFrame &frame);
private:
QCamera *m_camera;
QCameraImageCapture *m_capture;
QCameraViewfinder *m_viewfinder;
};
Camera::Camera(QObject *parent)
: QObject(parent)
{
m_camera = new QCamera(this);
m_capture = new QCameraImageCapture(m_camera, this);
m_viewfinder = new QCameraViewfinder(this);
m_camera->setViewfinder(m_viewfinder);
m_camera->setCaptureMode(QCamera::CaptureStillImage);
connect(m_camera, SIGNAL(stateChanged(QCamera::State)), this, SLOT(handleStateChanged(QCamera::State)));
connect(m_capture, SIGNAL(imageCaptured(int,QImage)), this, SIGNAL(imageCaptured(QImage)));
}
Camera::~Camera()
{
delete m_camera;
delete m_capture;
delete m_viewfinder;
}
void Camera::start()
{
m_camera->start();
}
void Camera::stop()
{
m_camera->stop();
}
void Camera::handleStateChanged(QCamera::State state)
{
if (state == QCamera::ActiveState) {
m_capture->capture();
}
}
void Camera::handleCapture(const QVideoFrame &frame)
{
QImage image = QImage(frame.bits(), frame.width(), frame.height(), QVideoFrame::imageFormatFromPixelFormat(frame.pixelFormat()));
emit imageCaptured(image);
}
```
这个代码示例创建了一个名为 `Camera` 的类,它使用了 Qt 的多媒体框架来调用摄像头并捕获图像。在 `start()` 方法中,它启动了摄像头,并在状态变为 `ActiveState` 时触发 `handleStateChanged()` 方法,该方法调用 `m_capture->capture()` 捕获图像。在 `handleCapture()` 方法中,它将捕获的图像转换为 `QImage` 格式,并通过信号 `imageCaptured()` 发出。
你可以在你的 Qt 应用程序中创建一个 `Camera` 对象,并连接到它的 `imageCaptured()` 信号以获取捕获的图像数据。你还可以使用 `m_viewfinder` 对象来显示摄像头捕获的实时图像。
注意,使用 Qt 的多媒体框架来调用 V4L2 可能需要一些额外的配置和调整,具体取决于你的摄像头和系统配置。你可能需要在项目文件中添加 `multimedia` 模块,并在代码中添加相关的头文件和库文件。
阅读全文
相关推荐
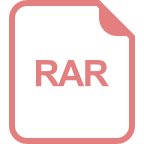
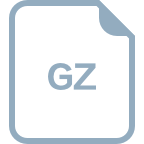
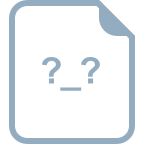
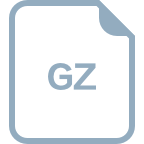
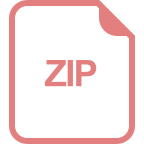
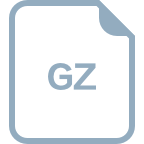
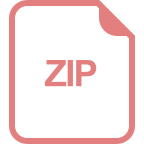

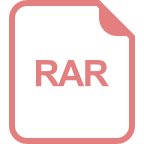
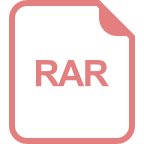
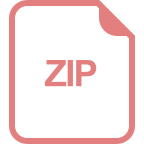
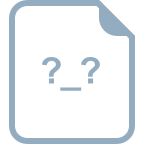
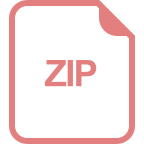
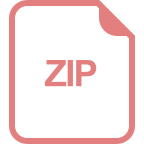
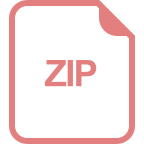

