定义学生类Student,其属性有姓名、出生年月(datetime)、年龄、性别(boolean)、爱好(list每个人有多个爱好),请利用Spring容器,来完成Student对象的管理,能够利用构造注入和Set注入的方式为其属性赋值。写出测试程序。
时间: 2024-10-11 13:11:08 浏览: 28
首先,我们创建一个`Student`类,包含所需的属性:
```java
import java.util.Date;
import java.util.List;
public class Student {
private String name;
private Date birthDate;
private int age;
private boolean gender;
private List<String> hobbies;
// getters and setters
public Student(String name, Date birthDate, int age, boolean gender, List<String> hobbies) {
this.name = name;
this.birthDate = birthDate;
this.age = age;
this.gender = gender;
this.hobbies = hobbies;
}
// toString method for testing purposes
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", birthDate=" + birthDate +
", age=" + age +
", gender=" + gender +
", hobbies=" + hobbies +
'}';
}
}
```
接下来,我们将`Student`添加到Spring的`ApplicationContext`中,通过构造函数注入和Set注入:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringTest {
private static ApplicationContext context;
@Autowired
public SpringTest(ApplicationContext applicationContext) {
context = applicationContext;
}
public void manageStudents() {
Student student1 = (Student) context.getBean("student1", Student.class);
System.out.println(student1); // 使用构造函数注入,假设XML配置中有名为"student1"的bean
Set<Student> students = (Set<Student>) context.getBeansOfType(Student.class).values();
for (Student student : students) {
System.out.println(student); // 使用Set注入
}
}
public static void main(String[] args) {
SpringTest test = new SpringTest(new ClassPathXmlApplicationContext("applicationContext.xml"));
test.manageStudents();
}
}
```
在这个例子中,你需要在`applicationContext.xml`文件中定义两个`<bean>`标签,分别为`student1`并设置其属性,以及一个`<set>`元素,用于存储多个`Student`实例。
测试程序会打印出每个`Student`对象的详细信息。
阅读全文
相关推荐
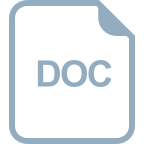
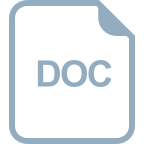
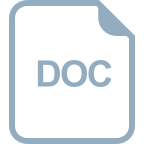

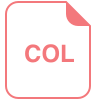
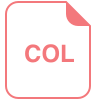
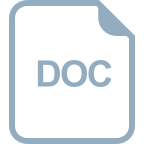
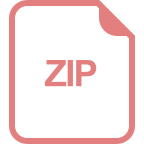
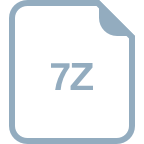
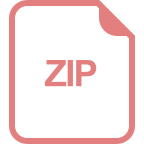
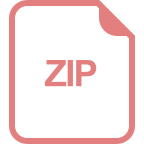